Point Labels
The point labels options are used to configure the point labels that are shown on the perimeter of the scale. They can be found in the pointLabels sub options object. Note
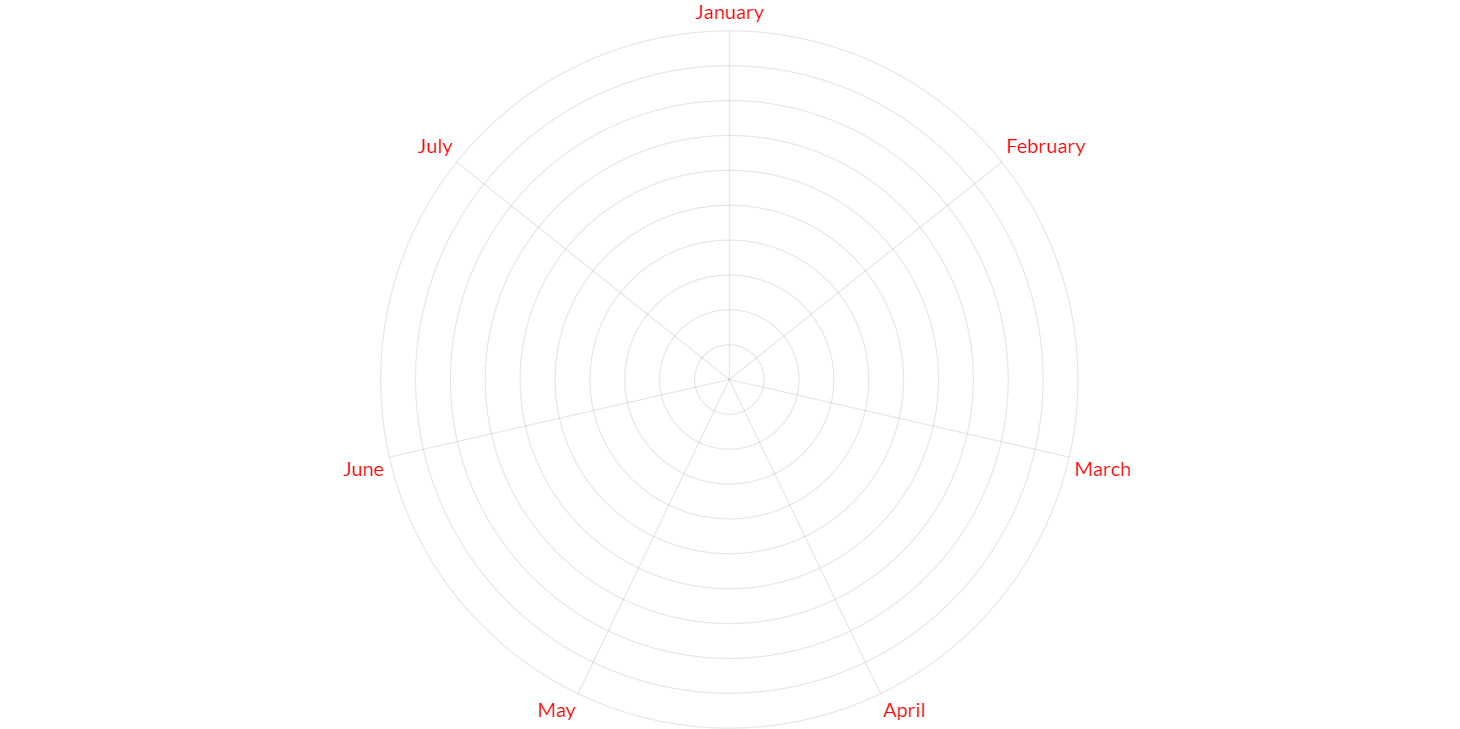
The point labels can be ONLY applied to radial axes.
These options only apply if display is true.
The RadialPointLabels provides all set and get methods to manage the configuration, as following:
// creates a radial axis
RadialAxis axis = new RadialAxis(chart);
// enables point labels
axis.getPointLabels().setDisplay(true);
// sets and gets the color value
axis.getPointLabels().setColor(HtmlColor.RED);
IsColor color = axis.getPointLabels().getColor();
Table with options:
Name | Type | Scriptable | Description |
---|---|---|---|
backdropColor | String - IsColor | Yes | Background color of the point label. |
backdropPadding | Padding | - | The padding of label backdrop. See padding documentation for more details. |
borderRadius | int - BarBorderRadius | Yes | The border radius of the point label (in pixels). |
centerPointLabels | boolean | - | If true , point labels are centered. |
display | boolean - Display | - | If true , point labels are shown. When Display.AUTO, the label is hidden if it overlaps with another label. |
color | String - IsColor | Yes | Color of point labels. |
font | IsFont | Yes | Font of point labels. |
padding | int | Yes | Padding between chart and point labels, in pixel. |
The further customization of point labels, a callback is provided.
Scriptable
Scriptable options at grid level accept a callback which is called for each of the underlying data values. See more details in Configuring charts section.
All scriptable options callbacks will get a ScaleContext instance.
// creates a radial axis
RadialAxis axis = new RadialAxis(chart);
// enables point labels
axis.getPointLabels().setDisplay(true);
// sets the option by a callback
axis.getPointLabels().setColor(new ColorCallback<ScaleContext>(){
@Override
public IsColor invoke(ScaleContext context){
// logic
return color;
}
});
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
backdropColor | ColorCallback<ScaleContext> | String - IsColor |
borderRadius | BorderRadiusCallback<ScaleContext> | int - BarBorderRadius |
color | ColorCallback<ScaleContext> | String - IsColor |
font | FontCallback<ScaleContext> | FontItem |
padding | SimplePaddingCallback | int |
Callback
Callback implementation can transform data labels to point labels. The default implementation simply returns the current string.
To apply an own callback, you can set a PointLabelCallback instance to the axis options, as following:
// creates a radial axis
RadialAxis axis = new RadialAxis(chart);
// enables point labels
axis.getPointLabels().setDisplay(true);
// sets callback
axis.getPointLabels().setCallBack(new PointLabelCallback(){
/**
* Callback function to transform data labels to point labels. The default implementation simply returns the current string.
*
* @param axis axis instance where this callback as been defined
* @param item label of current label
* @param index index of the label
* @return new label to apply to point label
*/
@Override
public String onCallback(Axis axis, String item, int index){
// logic
return item;
}
});
The callback can return a string (for single line) or a list of strings (for multiple lines).