Gradients
A gradient is a special type of image that consists of a progressive transition between two or more colors.
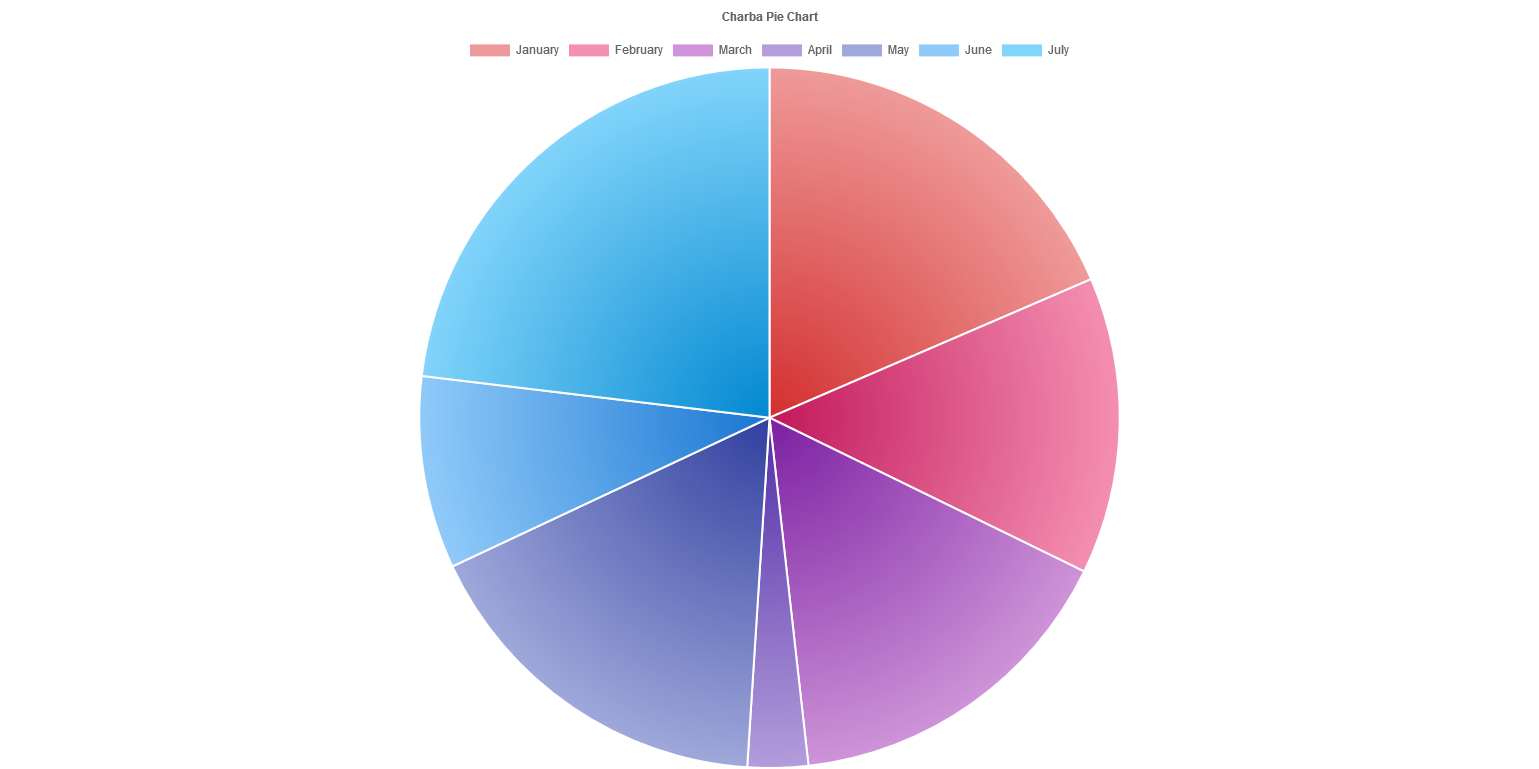
The gradient data type is defined with one of the types listed below.
- Linear where transitions colors progressively along an imaginary line.
- Radial where transitions colors progressively from a center point (origin).
As with any interpolation involving colors, gradients are calculated in the alpha-premultiplied color space. This prevents unexpected shades of gray from appearing when both the color and the opacity are changing.
Charba enables the usage of gradient providing some helpful configuration in order to enable you to do not use any dimensions or calculate them.
The gradient is mapped in the Gradient, which can configure both linear and radial gradients.
A Gradient can be created only by a gradient builder in order to optimize the cache used for them.
// creates a dataset
LineDataset dataset = new LineDataset();
// creates a gradient
// setting the 2 colors and their offset
Gradient gradient = GradientBuilder.create(GradientType.LINEAR, GradientScope.CHART).
addColorStop(0, HtmlColor.ORANGE).addColorStop(1, HtmlColor.PURPLE).build();
// sets the gradient as background color of the dataset
dataset.setBackgroundColor(gradient);
// fills the dataset
dataset.setFill(Fill.origin);
Types
Every gradient must be created setting which type represents. The gradient type must be passed to constructor of new gradient. If omitted, the default is linear.
Orientation
The Charba gradient implementation doesn't provide you to define the imaginary line or the direction from center but provides you a predefined sets of value, which can be used to create the canvas gradient.
The orientation values of the imaginary line or the direction from/to center are defined in an enumeration, gradient orientation.
Name | Type | Description | |
---|---|---|---|
TOP_DOWN | LINEAR | From top to bottom (vertical) | ![]() |
BOTTOM_UP | LINEAR | From bottom to to (vertical) | ![]() |
LEFT_RIGHT | LINEAR | From left to right (horizontal) | ![]() |
RIGHT_LEFT | LINEAR | From right to left (horizontal) | ![]() |
TOP_RIGHT | LINEAR | From top(left) to right(bottom) (diagonal) | ![]() |
BOTTOM_LEFT | LINEAR | From bottom(right) to left(top) (diagonal) | ![]() |
TOP_LEFT | LINEAR | From top(right) to left(bottom) (diagonal) | ![]() |
BOTTOM_RIGHT | LINEAR | From bottom(left) to right(top) (diagonal) | ![]() |
IN_OUT | RADIAL | From center to the borders | ![]() |
OUT_IN | RADIAL | From borders to the center | ![]() |
Scope
The Charba gradient implementation doesn't provide you to define the imaginary line or the direction from center but provides you a predefined sets of value, which can be used to create the canvas gradient.
The dimension of the area to use to calculate the gradient are defined in an enumeration, gradient scope.
Name | Description | |
---|---|---|
CANVAS | Uses the whole dimension of canvas | ![]() |
CHART | Uses the whole dimension of chart | ![]() |
Adding colors
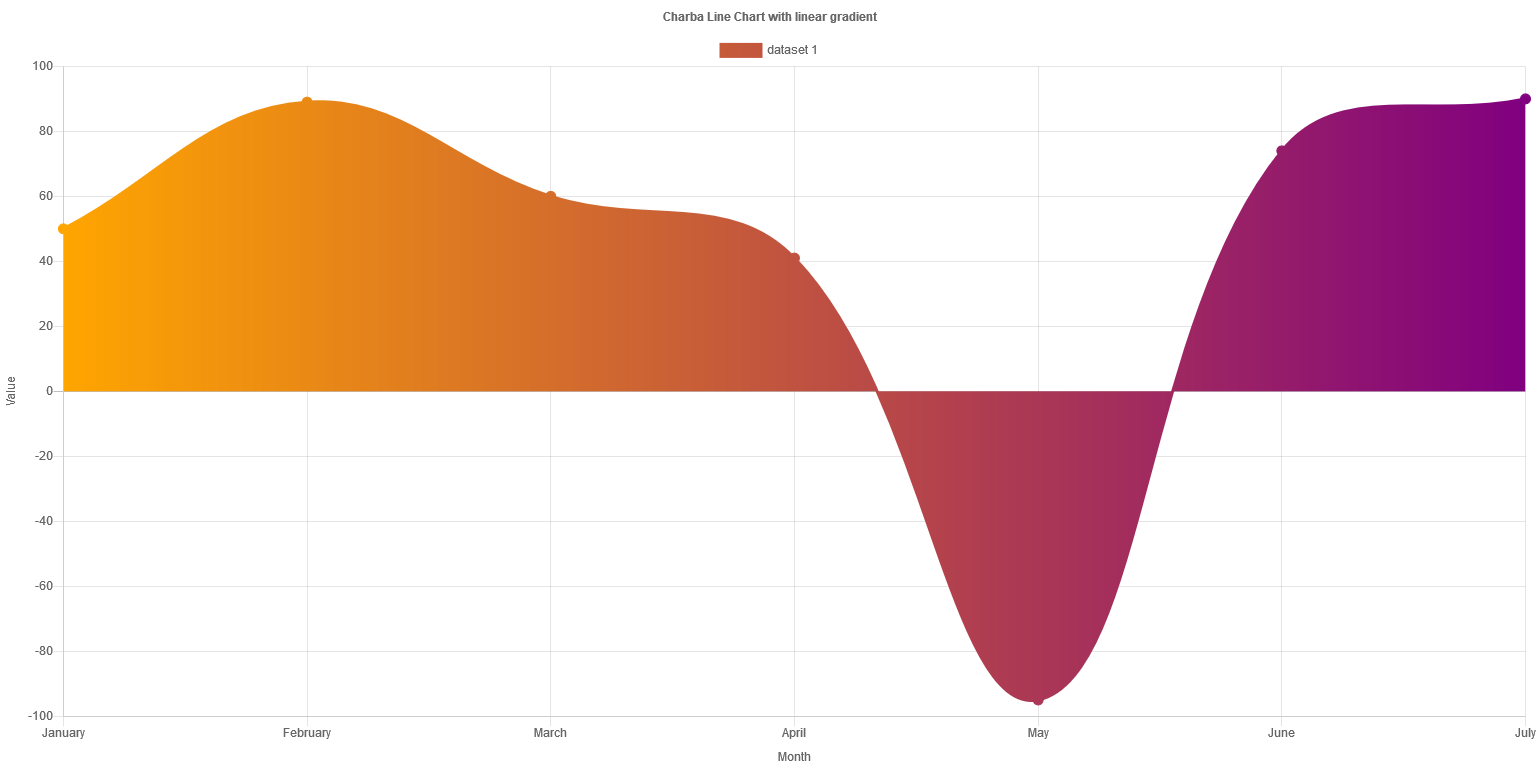
To complete a gradient configuration, you need to set a list of colors, adds a new color stop to the gradient, setting the offset, as value between 0 and 1 for where the color stop is located, and the color at the stop.
The gradient builder provides the methods to add colors, building the Gradient, as following:
// creates a gradient
// setting the 2 colors and their offset
Gradient gradient = GradientBuilder.create(GradientType.LINEAR, GradientScope.CHART)
.addColorStop(0, HtmlColor.ORANGE)
.addColorStop(0.5, HtmlColor.YELLOW)
.addColorStop(1, HtmlColor.PURPLE).build();
// creates a gradient
// setting the 2 colors directly
// with offset 0 and 1
Gradient gradient = GradientBuilder.create(GradientType.RADIAL, GradientScope.CANVAS)
.addColorsStartStop(HtmlColor.ORANGE, HtmlColor.PURPLE).build();
Resizing
Charba recalculates the gradients every time the dimension of chart or canvas occurs. In this way it maintains always the gradients even if the chart has got a different size comparing with it at creation time.