Patterns
A pattern represents an opaque object, based on an image, that it can be used to fill or stroke properties of a dataset chart.
Apart of the image, to create a pattern you could decide how to repeat the pattern's image. Possible values are:
- repeat (both directions)
- repeat-x (horizontal only)
- repeat-y (vertical only)
- no-repeat (neither direction)
If repetition is not specified creating a pattern, a value of repeat will be used.
A pattern is an alternative option to configure a dataset by Pattern object, instead of using a color.
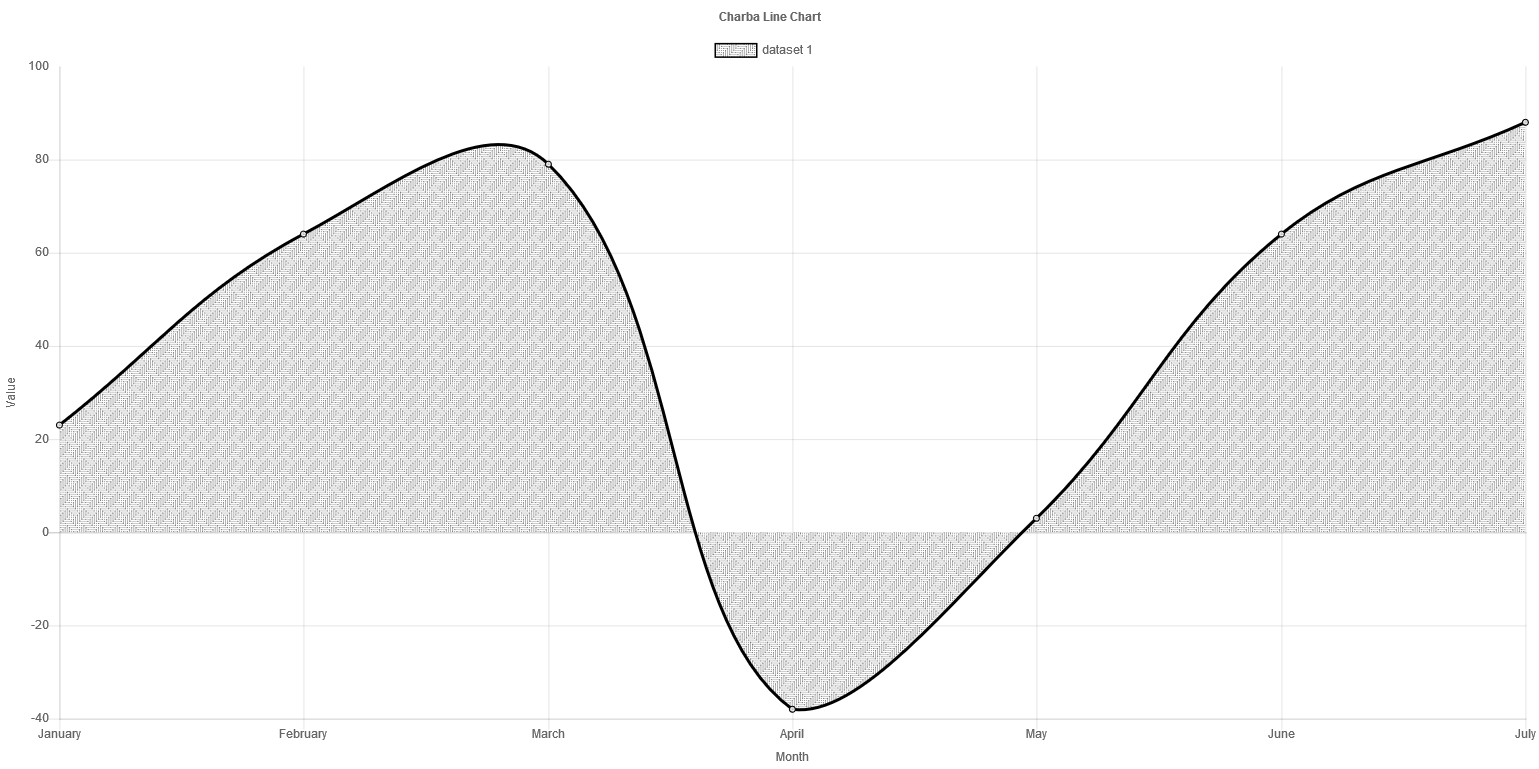
A Pattern can be created only by a patter builder in order to optimize the cache used for them.
For example, if you wanted to fill a dataset with a pattern from an image, you could do the following:
// creates a dataset
LineDataset dataset = new LineDataset();
// converts a "ImageResource" in "Img" class
Img img = ImagesHelper.toImg(Images.INSTANCE.pattern());
// creates a pattern by the image
Pattern pattern = PatternBuilder.create(img).build();
// sets the pattern as background color of the dataset
dataset.setBackgroundColor(pattern);
// fills the dataset
dataset.setFill(Fill.origin);
where Images.INSTANCE.pattern()
is a method of a ClientBundle, where the image is inside the project and using the ImagesHelper can be cast of a Img, as following:
public interface Images extends ClientBundle {
Images INSTANCE = GWT.create(Images.class);
@Source("/images/crossline-lines.png")
ImageResource pattern();
}
To avoid to have patterns without loaded images, it's suggested to prefetch the images at starting of application, leveraging on GWT Image as following:
// loads images
Image.prefetch(Images.INSTANCE.pattern().getSafeUri());
Tiles
Charba provides a tile builders which can easily generate canvas or Charba patterns with a set of shapes in order to use them in datasets, instead of colors.
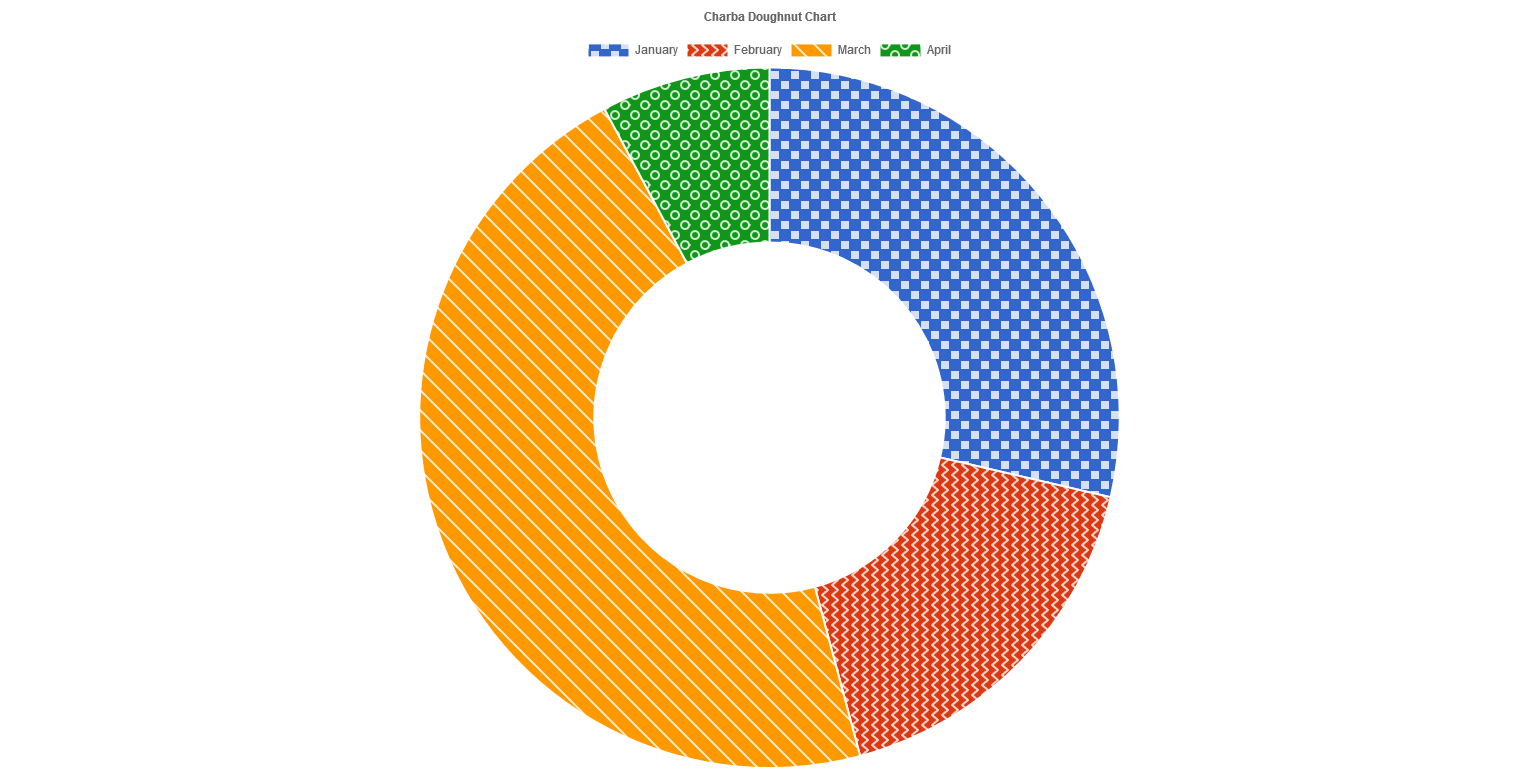
The main class to use this feature is TilesFactory which provides a set of methods to get a tile as canvas pattern or as Charba pattern which can be used in the datasets.
// creates a dataset
DoughnutDataset dataset = chart.newDataset();
// creates 4 tiles patterns for each data of the dataset
Pattern p1 = TilesFactory.createPattern(Shape.SQUARE, "#0000FF");
Pattern p2 = TilesFactory.createPattern(Shape.VERTICAL_ZIGZAG, "#FFFFFF");
Pattern p3 = TilesFactory.createPattern(Shape.DIAGONAL, "#FF0000");
Pattern p4 = TilesFactory.createPattern(Shape.RING, "#OOFFOO");
// sets the patterns as background color fo dataset
dataset.setBackgroundColor(p1, p2, p3, p4);
The tiles factory can also create canvas patterns to use wherever you want in your application:
// creates a DOM canvas pattern
CanvasPatternItem canvasPattern = TilesFactory.createTile(Shape.SQUARE, "#0000FF");
The tiles factory has got a default configuration which can be updated in order that new configuration can be used for all tiles.
The complete configuration items are described by following table:
Name | Type | Default | Description |
---|---|---|---|
shape | Shape | Shape.SQUARE | the shape to apply on tile |
backgroundColor | String - IsColor | rgba(100, 100, 100, 0.7) - | the background color of tile |
shapeColor | String - IsColor | rgba(255, 255, 255, 0.8) - | the shape color on tile |
size | int | 20 | the size of the tile (always a square). The minimum size for a tile is 10. |
lineCap | CapStyle | CapStyle.ROUND | determines the shape used to draw the end points of lines |
lineJoin | JoinStyle | JoinStyle.ROUND | determines the shape used to join two line segments where they meet |
This is the list of shapes, available out-of-the-box:
- back slashed line
- box
- cross
- cross dash
- dash
- diagonal
- diamond
- diamond box
- disc
- dot
- dot dash
- double diagonal
- empty star
- inverted diagonal
- inverted double diagonal
- inverted triangle
- line
- plus
- ring
- slashed line
- solid
- star
- square
- triangle
- weave
- zigzag
- vertical line
- vertical zigzag
Image shape
ImageShape enables to use an image and apply (scaling it) to the tile. The vantage is that we can decide the background of the image if it has got a transparent background.
ImageShape imageShape = new ImageShape(ImagesHelper.toImg(Images.INSTANCE.myImage()));
...
Pattern pattern = TilesFactory.createPattern(imageShape, "#990099");
...
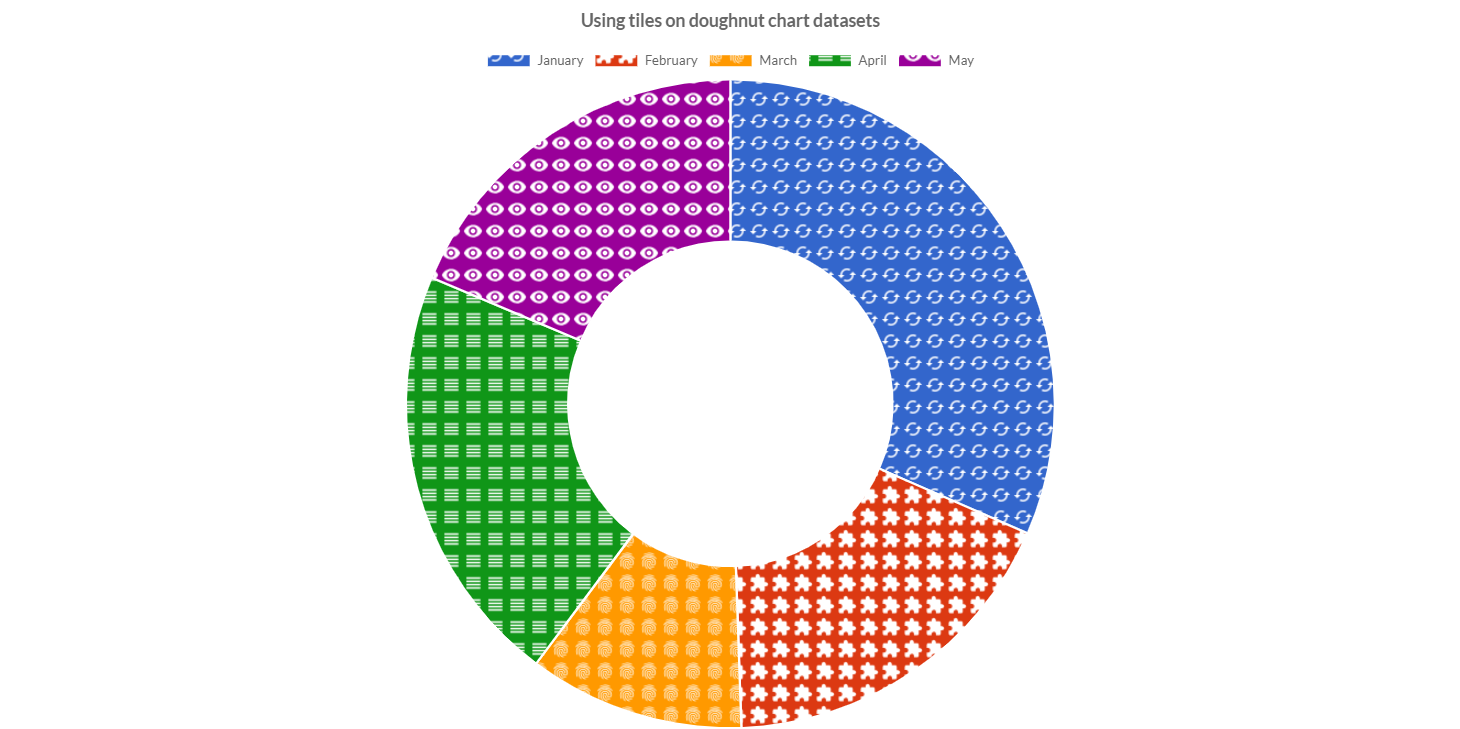
Character shape
CharacterShape enables to use a character (ONLY 1 otherwise you will get an exception) and apply to tile.
// creates a custom shape
CharacterShape charShape = new CharacterShape("m");
// creates a tile by the custom shape
Pattern pattern = TilesFactory.createPattern(charShape, "#990099");
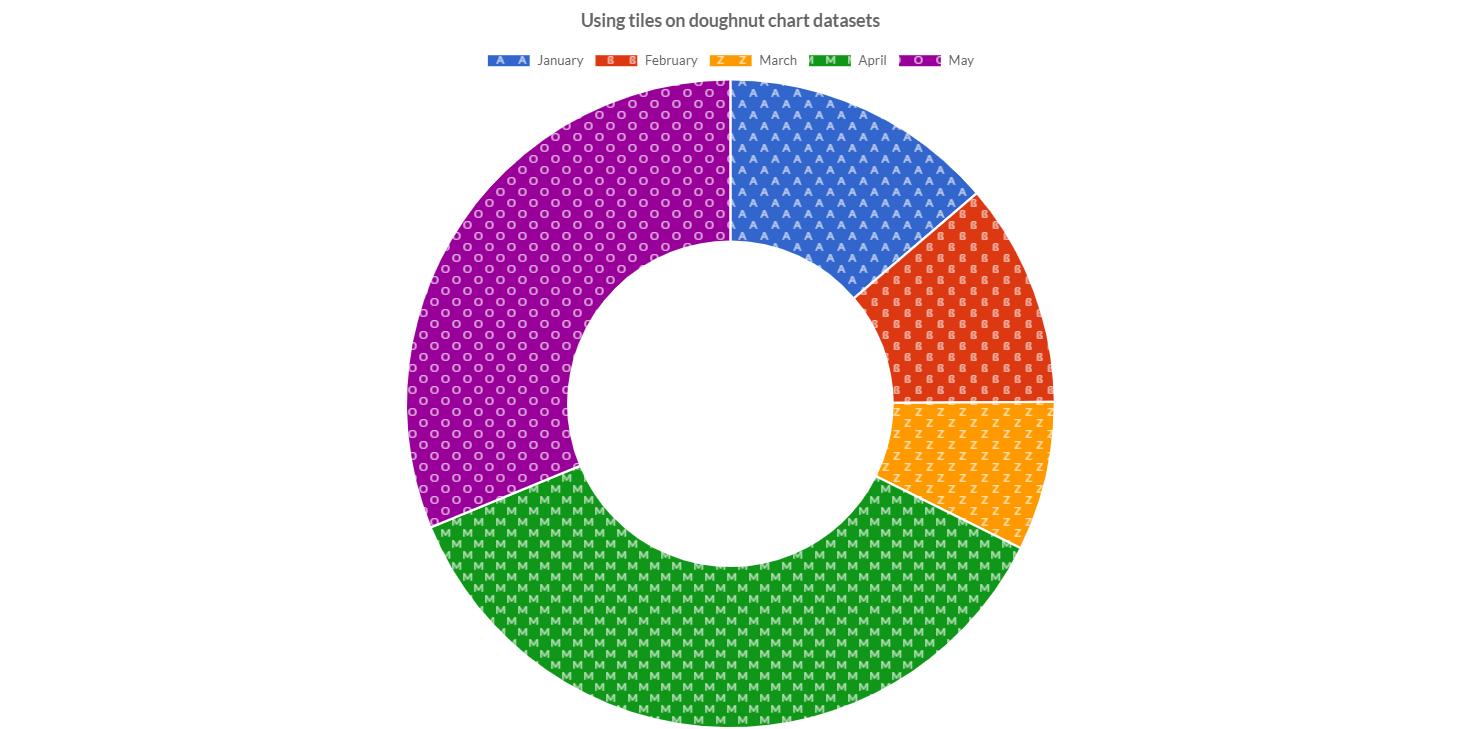
Point style shape
It is also possible to use PointStyle as shape for a tile.
// creates a shape by a point style
Pattern pattern = TilesFactory.createPattern(PointStyle.CIRCLE, "#990099");
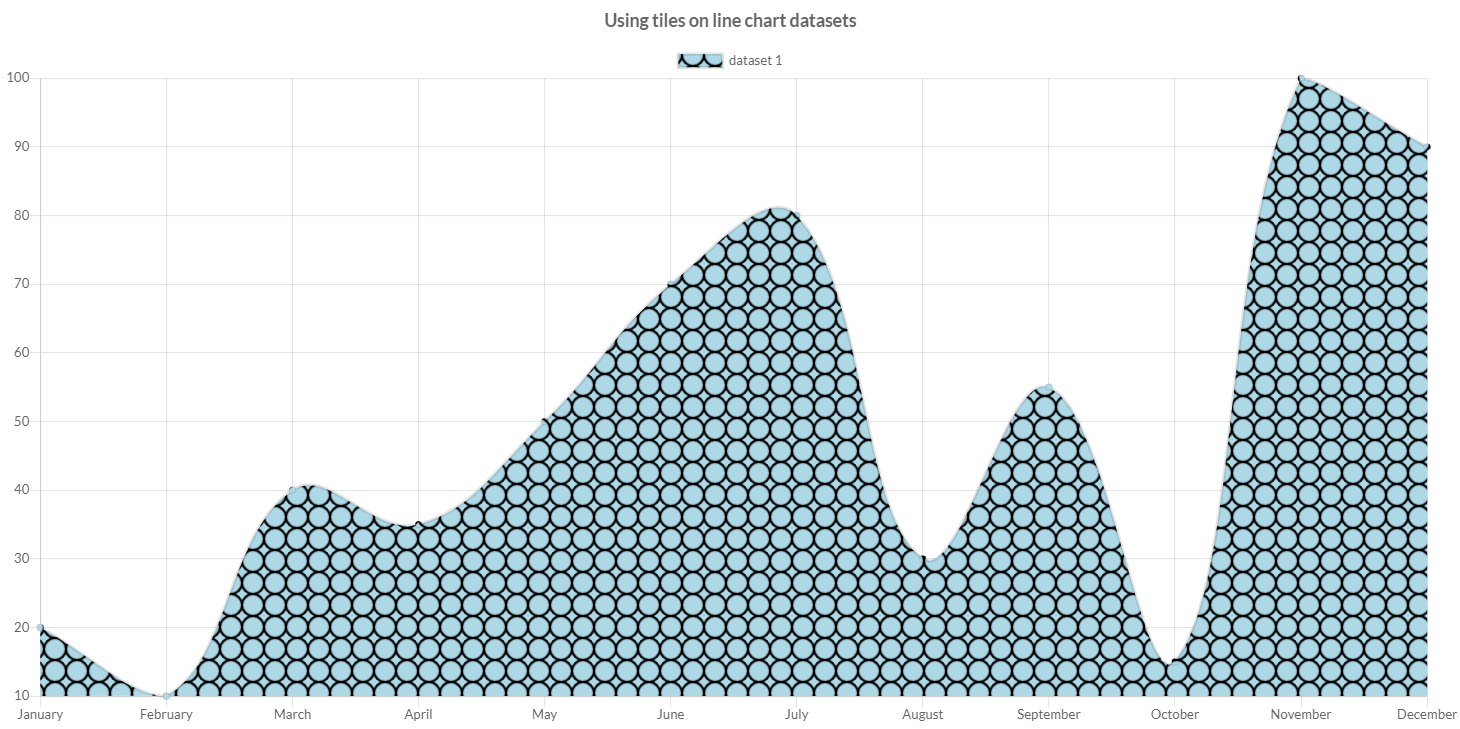
Builder
Charba provides a TilesBuilder to create tiles using the set methods in sequence and get a pattern or CanvasPatternItem at the end of configuration.
// creates a CHARBA pattern
Pattern pattern = TilesBuilder.create().setShape(Shape.EMPTY_STAR).setBackgroundColor("#990099").asPattern();
// creates a canvas pattern
CanvasPatternItem canvasPattern = TilesBuilder.create()
.setShape(Shape.EMPTY_STAR)
.setBackgroundColor("#990099")
.asTile();