Crosshair plugin
Charba provides a plugin implementation to set the crosshair on the chart when a chart.
Crosshairs are thin vertical and horizontal lines centered on a data point in a chart. When you, as a chart creator, enable crosshairs in your charts, your users will then be able to target a single element.
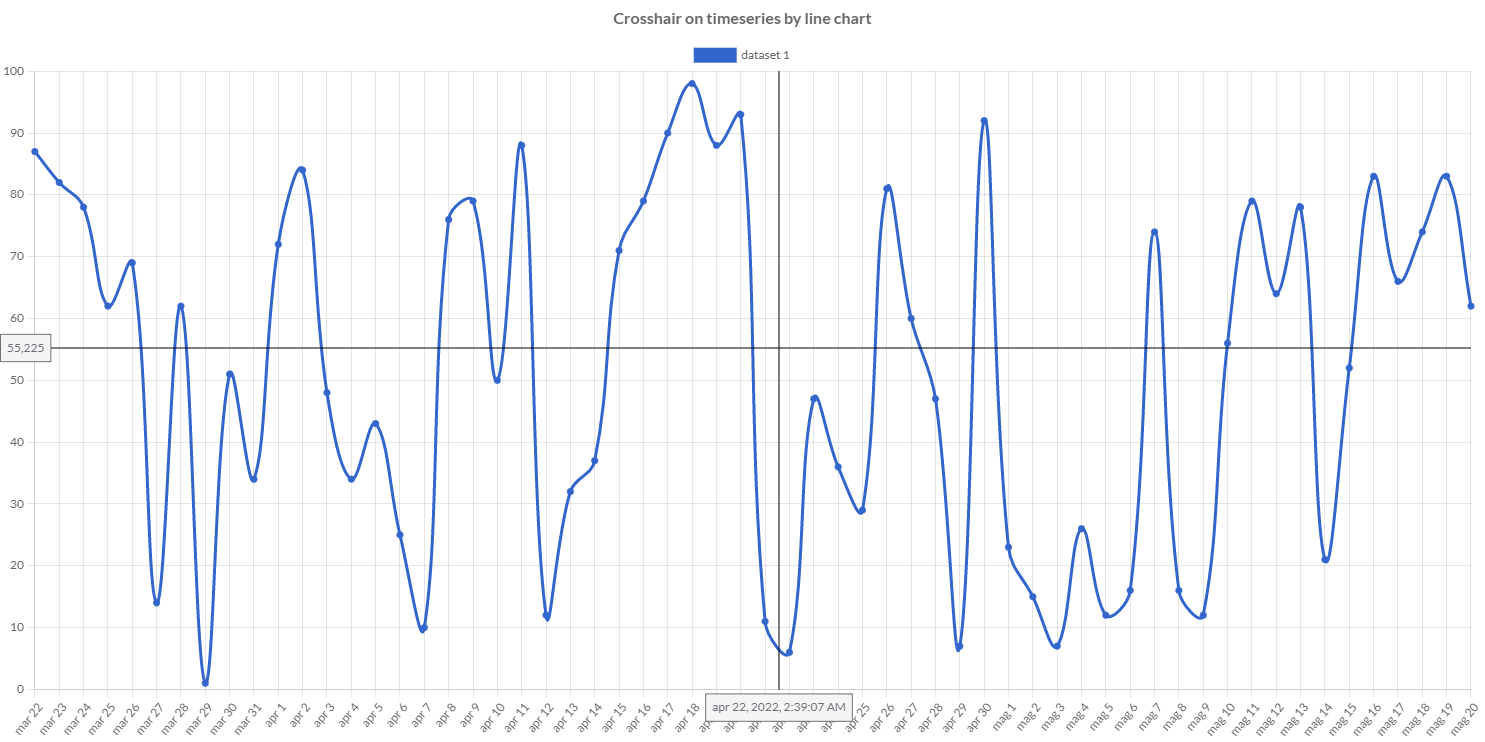
This plugin, as singleton, can be set both at global and at chart level.
The implementation is Crosshair and can be set as following:
// ---------------------------------
// Registers as global plugin
// ---------------------------------
Defaults.get().getPlugins().register(Crosshair.get());
// ---------------------------------
// Registers as single chart plugin
// ---------------------------------
chart.getPlugins().add(Crosshair.get());
The ID of plugin is charbacrosshair
(Crosshair.ID).
Options
It could be that you set this plugin as global one for all your charts but you want to change it for only one instance.
In this case you should instantiate a CrosshairOptions and set it to your chart options as following, setting the color you want:
// creates a plugin options
CrosshairOptions options = new CrosshairOptions();
// set mode
options.setMode(InteractionAxis.XY);
// --------------------------------------
// STORING plugin options
// --------------------------------------
// stores the plugin options by plugin ID
chart.getOptions().getPlugin().setOptions(Crosshair.ID, options);
// stores the plugin options without plugin ID
chart.getOptions().getPlugin().setOptions(options);
// stores the plugin options directly by the options
options.store(chart);
You can also change the default for all charts instances, as following
// creates a plugin options
CrosshairOptions options = new CrosshairOptions();
// set mode
options.setMode(InteractionAxis.XY);
// --------------------------------------
// STORING plugin options
// --------------------------------------
// stores the plugin options by plugin ID
Defaults.get().getGlobal().getPlugin().setOptions(Crosshair.ID, options);
// stores the plugin options without plugin ID
Defaults.get().getGlobal().getPlugin().setOptions(options);
// stores the plugin options directly by the options
options.store();
If you need to read the plugin options, there is the specific factory, CrosshairOptionsFactory as static reference inside the plugin which can be used to retrieve the options from chart, as following:
// gets options reference
CrosshairOptions options;
// --------------------------------------
// GETTING plugin options from chart
// --------------------------------------
if (chart.getOptions().getPlugin().hasOptions(Crosshair.ID)){
// retrieves the plugin options by plugin ID
options = chart.getOptions().getPlugin().getOptions(Crosshair.ID, Crosshair.FACTORY);
//retrieves the plugin options without plugin ID
options = chart.getOptions().getPlugin().getOptions(Crosshair.FACTORY);
}
The following are the attributes that you can set to plugin options:
Name | Type | Default | Description |
---|---|---|---|
enabled | boolean | true | If true , the plugin is enabled. |
group | String | null | Defines the group which the plugin instance belongs to. It can link the crosshair instances on multiple charts as group and the position is synchronized on all charts. |
lineColor | String - IsColor | HtmlColor.GRAY - | The color of the line of the cross hairs. |
lineDash | int[] | [2, 2] | The line dash pattern used when stroking lines, using an array of values which specify alternating lengths of lines and gaps which describe the pattern. |
lineDashOffset | double | 0 | Offset for line dashes. See MDN |
lineWidth | int | 1 | The width of the line of the cross hairs. |
mode | InteractionAxis | InteractionAxis.XY | Sets the display on horizontal and/or vertical hairs. |
modifierKey | ModifierKey | null | Keyboard modifier key which must be pressed to enable the cross hair. |
xScaleID | String - ScaleId | DefaultScaleId.X | The ID of the X axis to use to position the vertical cross hair. |
yScaleID | String - ScaleId | DefaultScaleId.Y | The ID of the Y axis to use to position the horizontal cross hair. |
Labelling
The plugin enables the labels on the scales in order to show the values of the cross hair. You can configure separately the labels on X and Y.
// creates a plugin options
CrosshairOptions options = new CrosshairOptions();
// set X label options
options.getXLabel().setBackgroundColor(HtmlColor.RED);
// set Y label options
options.getYLabel().setBackgroundColor(HtmlColor.YELLOW);
The following are the attributes that you can set to plugin label options:
Name | Type | Default | Description |
---|---|---|---|
display | boolean | true | If true , the label is shown. |
backgroundColor | String - IsColor | rgb(110, 112, 121) - | The background color of the label. |
borderColor | String - IsColor | HtmlColor.TRANSPARENT | The border color of the label. |
borderRadius | int | 6 | The border radius of the label. |
borderWidth | int | 0 | The border width of the label. |
color | String - IsColor | HtmlColor.WHITE - | The color of the text of the label. |
font | IsFont | See description | Font of the text of the label. The default value is the global font. See Font. |
padding | int | 6 | The space around the text of the label. |
To set the same value to both labels, without writing twice the same code, CrosshairOptions is providing a objet which enables that:
// creates a plugin options
CrosshairOptions options = new CrosshairOptions();
// set background color to X and Y labels
options.getLabels().setBackgroundColor(HtmlColor.RED);
Formatter
The plugin provides a callback to change the format the value of label text in order to show whatever is needed.
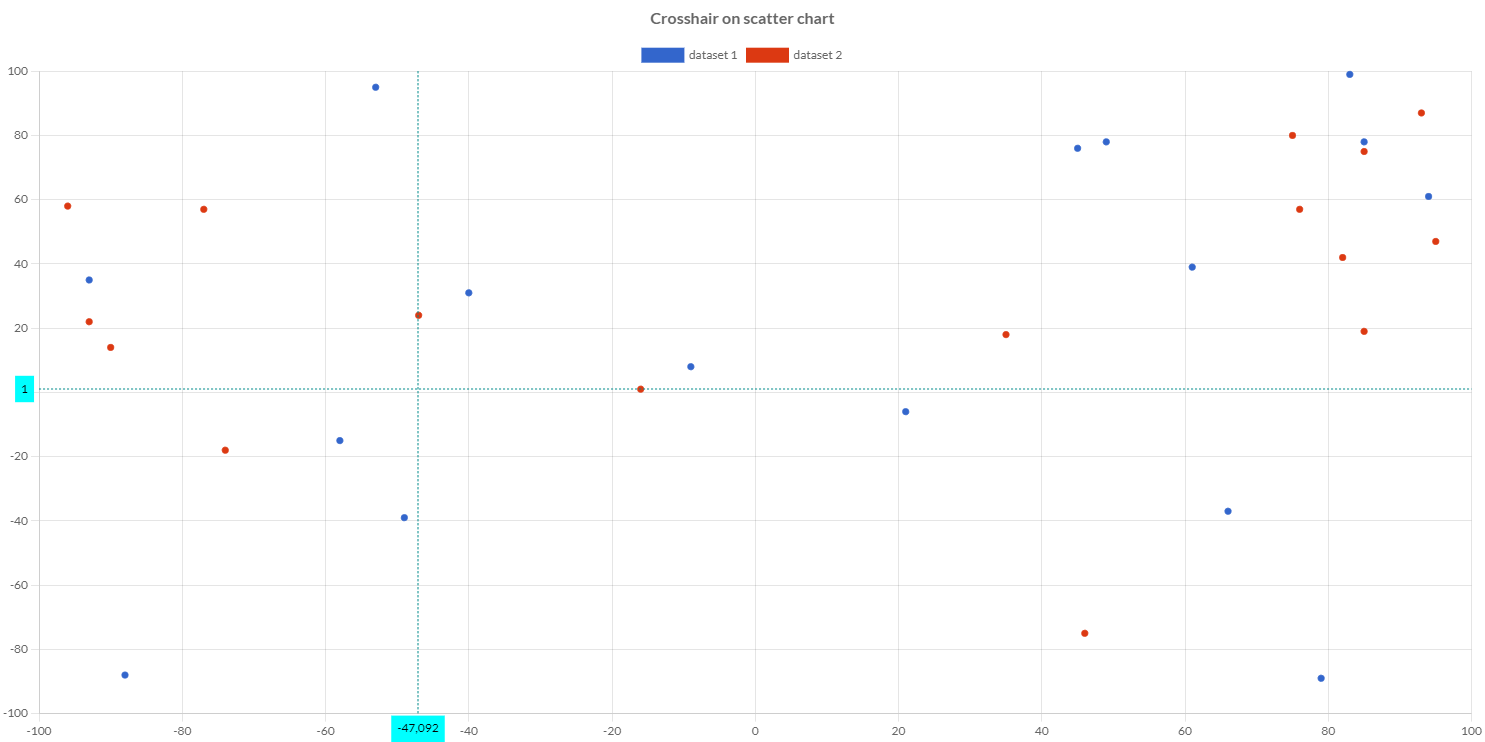
The formatter can be instantiated implementing CrosshairFormatterCallback interface which is providing 3 parameters:
- chart instance
- the scale instance, where the label is related to.
- the value, retrieved from the scale at the position of the cross hair.
// creates a plugin options
CrosshairOptions options = new CrosshairOptions();
// set Y label formatter
options.getYLabel().setFormatter(new CrosshairFormatterCallback() {
/**
* Returns the text to apply to the crosshair label.
*
* @param chart chart instance
* @param scale scale instance
* @param value current value to draw in the label
* @return the text to apply to the crosshair label
*/
@Override
public String format(IsChart chart, ScaleItem scale, ScaleValueItem value) {
if (format.getValue()) {
return Utilities.applyPrecision(value.getValue(), 0);
}
return value.getLabel();
}
});
Options builder
Charba provides a builder to create options using the set methods in sequence and get the options object at the end of configuration.
// creates a plugin options
CrosshairOptions options = CrosshairOptionsBuilder.create()
.setMode(InteractionAxis.XY)
.setXLabelBackgroundColor(HtmlColor.RED)
.build();
// sets options
chart.getOptions().getPlugins().setOptions(Crosshair.ID, options);
Creating a build by CrosshairOptionsBuilder.create()
it will use the global options as default, instead by CrosshairOptionsBuilder.create(chart)
it will use the global chart options as default.