Annotation plugin
Charba provides out of the box the feature to enable Annotation which can add annotations on a chart instance.
It can draw lines, boxes, points, labels, polygons and ellipses on the chart area.
The annotation plugin work with line, bar, scatter and bubble charts that use linear, logarithmic, time or category scales.
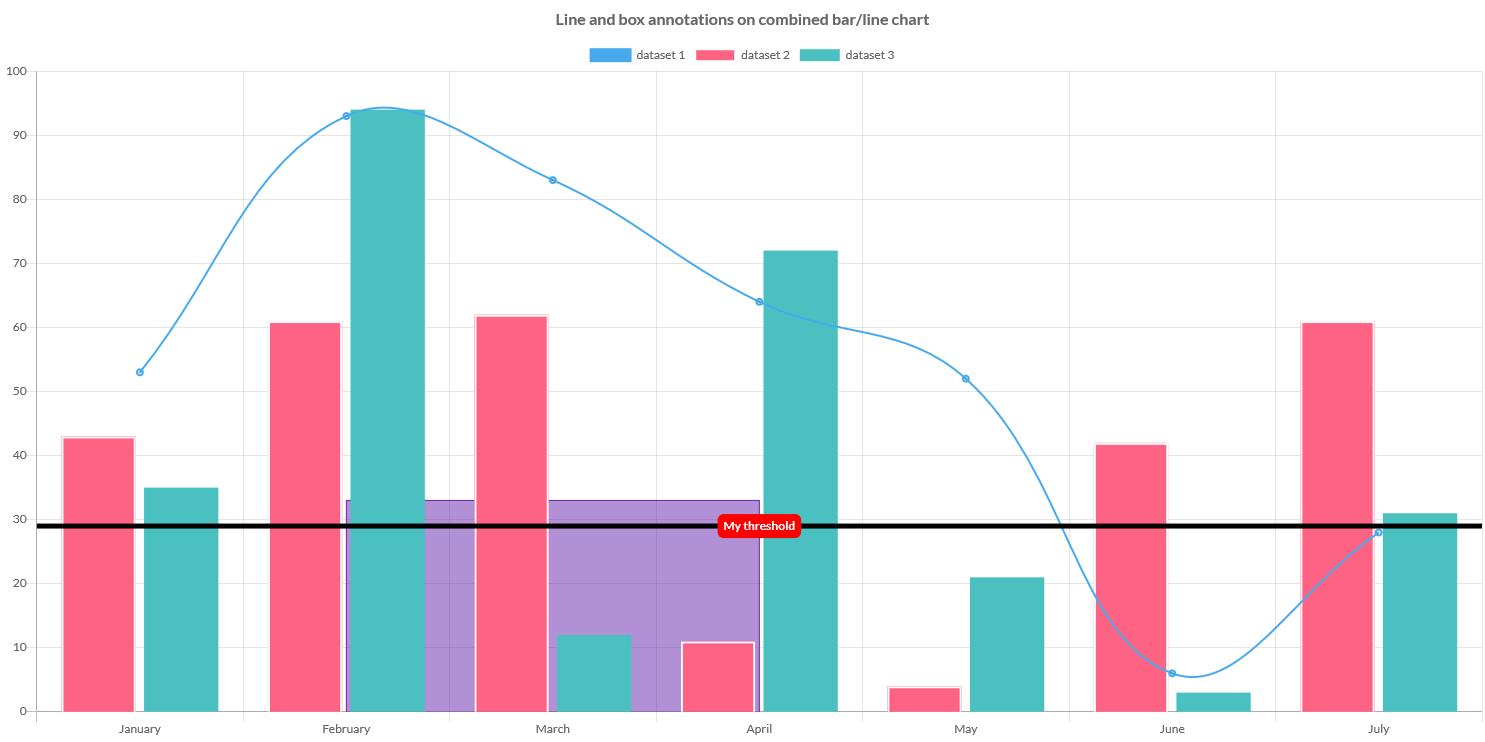
The annotation plugin will not work on any chart that does not have exactly two axes, including pie, radar and polar area charts.
Activation
The annotation plugin is injected directly in the document.
The plugin ID is a constant everywhere available, AnnotationPlugin.ID
, in AnnotationPlugin entry point.
This plugin registers itself globally, meaning that once injected, all charts will display annotations. In case you want it enabled only for a few charts, you can enable it as following:
// --------------------------------------
// enabling the plugin without any parameter
// the plugin is NOT registered to all charts
// --------------------------------------
AnnotationPlugin.enable();
// --------------------------------------
// enabling the plugin with `true` parameter
// the plugin is registered to all charts
// --------------------------------------
AnnotationPlugin.enable(true);
To activate the plugin in a specific chart, it's enough to provide the configuration options (see below) or enabling it by:
// --------------------------------------
// ENABLING the plugin to a chart instance
// storing a plugin options
// --------------------------------------
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// sets default draw time
options.setDrawTime(DrawTime.BEFORE_DRAW);
// stores the plugin options directly by the options
options.store(chart);
// --------------------------------------
// ENABLING the plugin to a chart instance
// by a boolean using default plugin
// options
// --------------------------------------
chart.getOptions().getPlugins().setEnabled(AnnotationPlugin.ID, true);
Configuration
The plugin options can be changed at 2 different levels and are evaluated with the following priority:
- per chart by
chart.getOptions().getPlugins().setOptions
method - or globally by
Defaults.get().getGlobal().getPlugins().setOptions
method
The configuration AnnotationOptions class is the entry point of plugin configuration.
// -------------------------
// CONFIGURES the annotation
// by AnnotationId id
// -------------------------
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LineAnnotation line = new LineAnnotation();
... // additional label configuration
// sets the line annotation to the options
options.setAnnotations(line);
// stores the plugin options directly by the options
options.store();
You can also change the default for all charts instances, as following:
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LineAnnotation line = new LineAnnotation();
... // additional label configuration
// sets the line annotation to the options
options.setAnnotations(line);
// --------------------------------------
// STORING plugin options
// --------------------------------------
// stores the plugin options by plugin ID
Defaults.get().getGlobal().getPlugin().setOptions(AnnotationPlugin.ID, options);
// stores the plugin options without plugin ID
Defaults.get().getGlobal().getPlugin().setOptions(options);
// stores the plugin options directly by the options
options.store();
If you need to read the plugin options, there is the specific factory, AnnotationOptionsFactory as static reference inside the AnnotationPlugin entry point which can be used to retrieve the options from chart as following:
// gets options reference
AnnotationOptions options;
// --------------------------------------
// GETTING plugin options from chart
// --------------------------------------
if (chart.getOptions().getPlugin().hasOptions(AnnotationPlugin.ID)){
// retrieves the plugin options by plugin ID
options = chart.getOptions().getPlugin().getOptions(AnnotationPlugin.ID, AnnotationPlugin.FACTORY);
//retrieves the plugin options without plugin ID
options = chart.getOptions().getPlugin().getOptions(AnnotationPlugin.FACTORY);
}
Default element options
Every annotation type is a chart element and the common options for a specific type can be configured by elements in the chart and global options:
- per chart by
chart.getOptions().getElements().getElement(ElementFactory)
method - or globally by
Defaults.get().getGlobal().getElements().getElement(ElementFactory)
method
The annotation options are providing a ElementFactory instance in order to enable to get and change the options at annotation type level.
// ----------------------------------------------------
// Setting option for all box annotations of the chart
// ----------------------------------------------------
BoxAnnotation boxElement = chart.getOptions().getElements().getElement(BoxAnnotation.FACTORY);
// checks if consistent
if (boxElement != null) {
// set font family for all box labels of the chart
boxElement.getLabel().getFont().setFamily("courier");
}
// ----------------------------------------------------
// Setting option for all box annotations
// ----------------------------------------------------
BoxAnnotation boxElement = Defaults.get().getGlobal().getElements().getElement(BoxAnnotation.FACTORY);
// checks if consistent
if (boxElement != null) {
// set font family for all box labels of all charts
boxElement.getLabel().getFont().setFamily("courier");
}
If the annotation plugin is not activated (see Activation), the annotation element options can not be set because the result will be always an inconsistent options instance.
Identifier
Every annotation configuration can be add to the AnnotationOptions, assigning a unique identifier.
The identifier of a label configuration can be set by a string or by a specific class, AnnotationId.
// -------------------------
// CONFIGURES the annotation
// by AnnotationId id
// -------------------------
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates annotation id
AnnotationId annotationId = AnnotationId.create("myAnnotation1");
// creates and adds a line annotation by "myAnnotation1" id
LineAnnotation line = new LineAnnotation(annotationId);
// configures the line annotation
line.setDrawTime(DrawTime.AFTER_DATASETS_DRAW);
line.setBorderColor(HtmlColor.BLACK);
line.setBorderWidth(5);
... // additional label configuration
// sets the line annotation to the options
options.setAnnotations(line);
// stores the plugin options directly by the options
options.store();
If the annotation id is not provided, a unique id for the annotation is created automatically, which can be retrieve by getId()
method of the annotation.
You can access to the configured annotations configurations as following:
// ------------------------
// GETS the configured
// annotations
// ------------------------
// retrieves the plugin options by plugin ID
AnnotationOptions options = chart.getOptions().getPlugin().getOptions(AnnotationPlugin.FACTORY);
// gets all annotations configurations
List<AbstractAnnotation> allAnnotations = options.getAnnotations();
// gets "myAnnotation1" annotation configuration
AbstractAnnotation annotation1 = options.getAnnotation(AnnotationId.create("myAnnotation1"));
// gets "myAnnotation2" annotation configuration
AbstractAnnotation annotation2 = options.getAnnotation("myAnnotation2");
Options
The configuration class AnnotationOptions contains all properties needed to configure the plugin.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// sets default draw time
options.setDrawTime(DrawTime.BEFORE_DRAW);
The following options are available at the top level. They apply to all annotations unless they are overwritten on a per-annotation basis:
Name | Type | Default | Description |
---|---|---|---|
animations | Animations | - | See animation options section for more details. |
clip | boolean | true | If true , the annotations are clipped to the chart area. |
drawTime | DrawTime | DrawTime.AFTER_DATASETS_DRAW | Defines when the annotations are drawn. This allows positioning of the annotation relative to the other elements of the graph. |
events | IsEvent[] - Set<IsEvent> | Defaults.get().getGlobal() .getEvents() | The events option defines the browser events that the plugin should listen to. This overrides the options at chart level. |
interaction | Interaction | see below | To configure which events trigger plugin interactions. |
Animations
Animations options configures which element properties are animated and how.
The animations element is a container of configurations which can be stored and retrieved by a key.
The following options are available in AnimationCollection.
Name | Type | Defaults | Description |
---|---|---|---|
delay | int | Undefined.INTEGER | Delay in milliseconds before starting the animations. |
duration | int | 1000 | The number of milliseconds an animation takes. |
easing | Easing | Easing.EASE_OUT_QUART | Easing function to use. See Robert Penner's easing equations for more details. |
interpolator | NativeInterpolator | - | Enables a custom interpolation during the animations. Only coding in java script for performance reasons. |
loop | boolean | false | If set to true , the animations loop endlessly. |
properties | String[] - Key[] | [] | The properties of elements to use to animate. |
type | AnimationType | AnimationType.NUMBER | Type of property, determines the interpolator used. |
from | boolean - double - String - IsColor | Undefined.BOOLEAN Undefined.DOUBLE null | Start value for the animation. |
to | boolean - double - String - IsColor | Undefined.BOOLEAN Undefined.DOUBLE null | End value for the animation. |
Interaction
Interaction uses the same format as a chart instance.
DefaultInteractionMode.INDEX
and DefaultInteractionMode.DATASET
modes are not supported by the plugin. If set, the plugin will use DefaultInteractionMode.NEAREST
mode.
InteractionAxis.R
is not supported by the plugin. If set, the plugin will use InteractionAxis.XY
mode.
Draw time
The DrawTime option for an annotation determines where in the chart life cycle the drawing occurs. Four potential options are available:
Option | Description |
---|---|
BEFORE_DRAW | Occurs before any drawing takes place |
BEFORE_DATASETS_DRAW | Occurs after drawing of axes, but before data sets |
AFTER_DATASETS_DRAW | Occurs after drawing of data sets but before items such as the tooltip |
AFTER_DRAW | After other drawing is completed. |
Box
Box annotations are used to draw rectangles on the chart area. This can be useful for highlighting different areas of a chart.
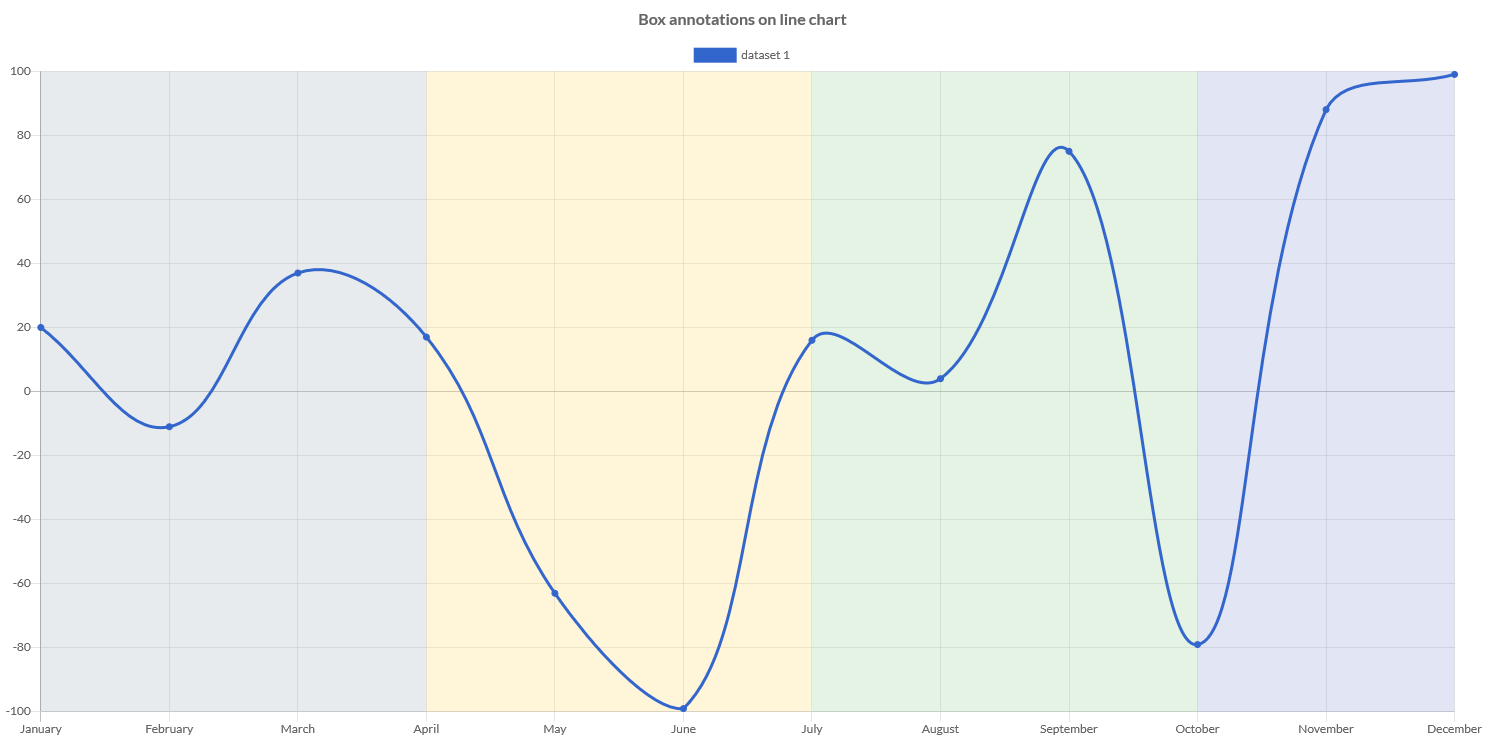
Every plugin options can have an unlimited number of boxes annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
BoxAnnotation box = new BoxAnnotation();
box.setDrawTime(DrawTime.BEFORE_DATASETS_DRAW);
box.setXScaleID(DefaultScaleId.X);
box.setYScaleID(DefaultScaleId.Y);
box.setXMin("January");
box.setXMax("April");
box.setBackgroundColor(GwtMaterialColor.YELLOW_LIGHTEN_3.alpha(0.3D));
box.setBorderWidth(0);
// stores the annotation in the main options
options.setAnnotations(box);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
adjustScaleRange | boolean | true | Yes | If true , the scale range should be adjusted if this annotation is out of range. |
backgroundColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The fill color of the box. See default colors. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderCapStyle | CapStyle | CapStyle.BUTT | Yes | Cap style of the border line. See MDN. |
borderColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The stroke color of the box. See default colors. |
borderDash | int[] | [] | Yes | The length and spacing of dashes. See MDN. |
borderDashOffset | double | 0 | Yes | The offset for line dashes. See MDN. |
borderJoinStyle | JoinStyle | JoinStyle.MITER | Yes | Border line joint style. See MDN |
borderRadius | int - BarBorderRadius | 0 | Yes | The radius in pixels of box rectangle. |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 1 | Yes | The stroke width of the box. |
display | boolean | true | Yes | Whether or not this annotation is visible. |
drawTime | DrawTime | DrawTime. AFTER_DATASETS_DRAW | Yes | Defines when the annotation is drawn. This allows positioning of the annotation relative to the other elements of the graph. |
init | boolean | false | - | If true or customized by callback, the annotation element will be animated since the initial phase. |
rotation | double | 0 | Yes | The rotation of annotation, in degrees. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
xMax | String - double - Date | null | Yes | Right edge of the box in units along the x axis. |
xMin | String - double - Date | null | Yes | Left edge of the box in units along the x axis. |
xScaleID | String - ScaleId | null | - | The ID of the X scale to bind onto. |
yMax | String - double - Date | null | Yes | Top edge of the box in units along the y axis. |
yMin | String - double - Date | null | Yes | Bottom edge of the box in units along the y axis. |
yScaleID | String - ScaleId | null | - | The ID of the Y scale to bind onto. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
If one of the axes does not match an axis in the chart, the box will take the entire chart dimension.
The 4 coordinates, xMin, xMax, yMin, yMax are optional. If not specified, the box is expanded out to the edges in the respective direction.
Box scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
BoxAnnotation box = new BoxAnnotation();
// sets callback for border width options
box.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(box);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
adjustScaleRange | AdjustScaleRangeCallback | boolean |
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderCapStyle | CapStyleCallback<AnnotationContext> | CapStyle |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderJoinStyle | JoinStyleCallback<AnnotationContext> | JoinStyle |
borderRadius | BorderRadiusCallback<AnnotationContext> | int - - BarBorderRadius |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
drawTime | DrawTimeCallback | DrawTime |
rotation | RotationCallback<AnnotationContext> | double |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
xMax | ValueCallback | String - double - Date |
xMin | ValueCallback | String - double - Date |
yMax | ValueCallback | String - double - Date |
yMin | ValueCallback | String - double - Date |
z | ZCallback | int |
Box label
A box annotation can have a label to draw in the box.
Every box annotation can have ONLY 1 label.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
BoxAnnotation box = new BoxAnnotation();
// sets label configuration
box.getLabel().setDisplay(true);
box.getLabel().setContent("My threshold");
// stores the annotation in the main options
options.setAnnotations(box);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
color | String[] - IsColor[] | #fff - | Yes | The text color of the label. |
content | String - String[] - Img - Canvas | null | Yes | The content to show in the label. Provide an array to display values on a new line. |
display | boolean | false | Yes | Whether or not the label is shown. |
drawTime | DrawTime | See description | - | Defines when the label is drawn. Defaults to the box annotation draw time if unset. |
font | IsFont - List<FontItem> - FontItem[] | See description | Yes | The text font of the label. The default value is the global font with the weight set to Weight.BOLD. See Font. |
imageHeight | int - String | Undefined.INTEGER - null | Yes | Overrides the height of the image. Could be set in pixel by a number, or in percentage of current height of image by a string. If uset, uses the height of the image. It is used only when the content is an image. |
imageOpacity | double | 0 | Yes | Overrides the opacity of the image or canvas element. Could be set a number in the range 0.0 to 1.0, inclusive. It is used only when the content is an image or canvas element. |
imageWidth | int - String | Undefined.INTEGER - null | Yes | Overrides the width of the image. Could be set in pixel by a number, or in percentage of current width of image by a string. If unset, uses the width of the image. It is used only when the content is an image. |
padding | Padding | 6 | Yes | Number of pixels to add above and below the title text. See padding documentation for more details. |
position | AlignPosition | See position | Yes | Anchor position of label on box. |
rotation | double | 0 | Yes | The rotation of label, in degrees. |
textAlign | TextAlign | TextAlign.START | Yes | Horizontal alignment on the label content when is set as a multiple lines text. |
textStrokeColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The stroke color of the text. |
textStrokeWidth | int | 0 | Yes | The stroke width of the text. |
xAdjust | double | 0 | Yes | Adjustment along x-axis (left-right) of label relative to computed position. Negative values move the label left, positive right. |
yAdjust | double | 0 | Yes | Adjustment along y-axis (top-bottom) of label relative to computed position. Negative values move the label up, positive down. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
Box label position
AlignPosition can define the x
property for the horizontal alignment in the box. Similarly, the y
property defines the vertical alignment in the box.
Possible options for both properties are LabelPosition.START, LabelPosition.CENTER, LabelPosition.END, or a double, a value between 0 and 1, is representing the percentage on the size where the label will be located.
Default is LabelPosition.CENTER.
Box label scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
BoxAnnotation box = new BoxAnnotation();
// sets callback for color options
box.getLabel().setColor(new ColorCallback<AnnotationContext>(){
@Override
public IsColor invoke(AnnotationContext context){
// logic
return color;
}
});
// stores the annotation in the main options
options.setAnnotations(box);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
color | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
content | ContentCallback | String - List<String> - Img - Canvas |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
font | FontsCallback<AnnotationContext> | List<FontItem> |
imageHeight | ImageSizeCallback | String - int |
imageOpacity | ImageOpacityCallback | double |
imageWidth | ImageSizeCallback | String - int |
padding | PaddingCallback<AnnotationContext> | PaddingItem |
rotation | RotationCallback<AnnotationContext> | double |
position | LabelAlignPositionCallback | AlignPosition |
textAlign | TextAlignCallback<AnnotationContext> | TextAlign |
textStrokeColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
textStrokeWidth | WidthCallback<AnnotationContext> | int |
xAdjust | AdjustSizeCallback | double |
yAdjust | AdjustSizeCallback | double |
z | ZCallback | int |
Ellipse
Ellipse annotations are used to draw ellipses on the chart area. This can be useful for highlighting different areas of a chart.
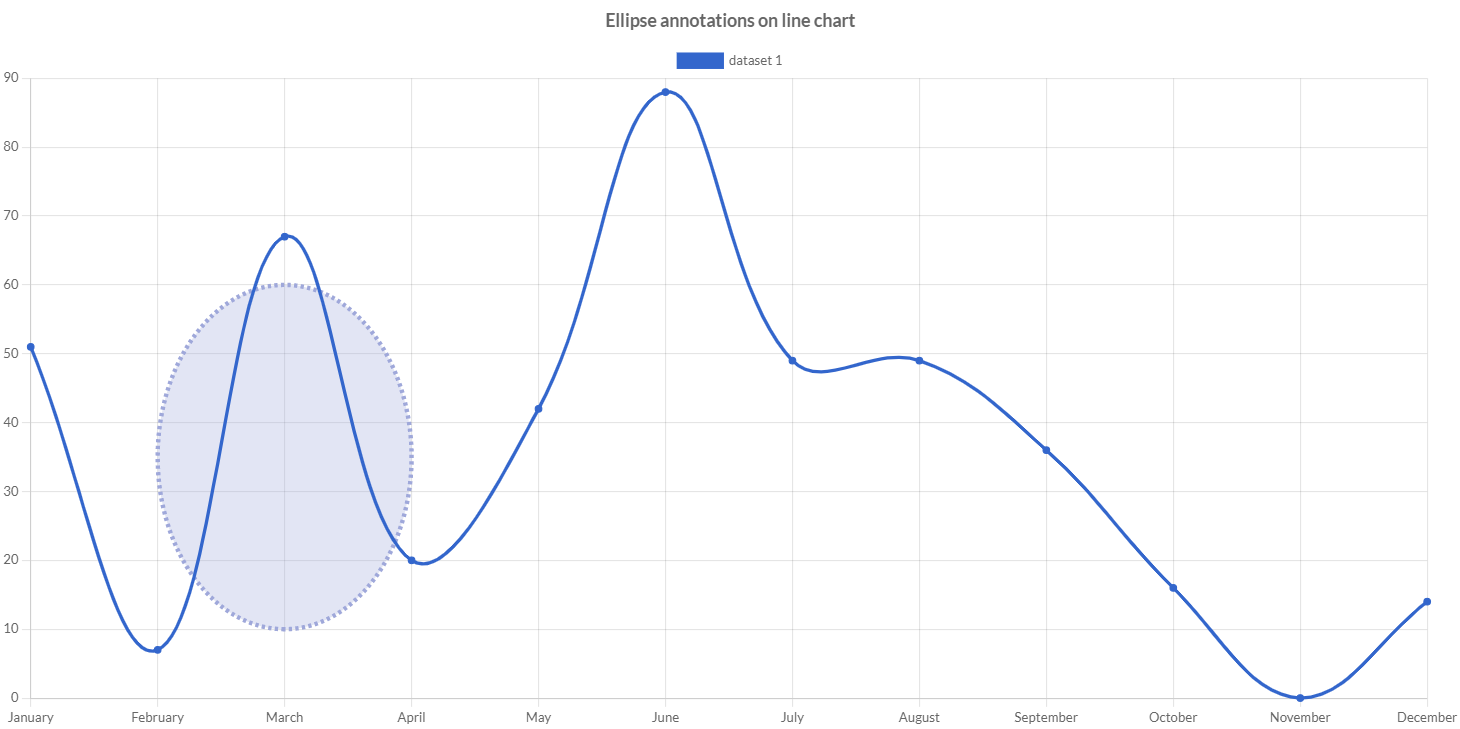
Every plugin options can have an unlimited number of ellipses annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
EllipseAnnotation ellipse = new EllipseAnnotation();
ellipse.setDrawTime(DrawTime.BEFORE_DATASETS_DRAW);
ellipse.setXScaleID(DefaultScaleId.X);
ellipse.setYScaleID(DefaultScaleId.Y);
ellipse.setXMin("February");
ellipse.setXMax("April");
ellipse.setYMin(10);
ellipse.setYMax(60);
ellipse.setBackgroundColor(GwtMaterialColor.INDIGO_LIGHTEN_3.alpha(0.3D));
ellipse.setBorderWidth(4);
ellipse.setBorderColor(GwtMaterialColor.INDIGO_LIGHTEN_3);
ellipse.setBorderDash(3,3);
// stores the annotation in the main options
options.setAnnotations(ellipse);
If one of the axes does not match an axis in the chart, the ellipse will take the entire chart dimension.
The 4 coordinates, xMin, xMax, yMin, yMax are optional. If not specified, the ellipse is expanded out to the edges in the respective direction.
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
adjustScaleRange | boolean | true | Yes | If true , the scale range should be adjusted if this annotation is out of range. |
backgroundColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The fill color of the ellipse. See default colors. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The stroke color of the ellipse. See default colors. |
borderDash | int[] | [] | Yes | The length and spacing of dashes. See MDN. |
borderDashOffset | double | 0 | Yes | The offset for line dashes. See MDN. |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 1 | Yes | The stroke width of the ellipse. |
display | boolean | true | Yes | Whether or not this annotation is visible. |
drawTime | DrawTime | DrawTime. AFTER_DATASETS_DRAW | Yes | Defines when the annotation is drawn. This allows positioning of the annotation relative to the other elements of the graph. |
init | boolean | false | - | If true or customized by callback, the annotation element will be animated since the initial phase. |
rotation | double | 0 | Yes | The rotation of annotation, in degrees. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
xMax | String - double - Date | null | Yes | Right edge of the ellipse in units along the x axis. |
xMin | String - double - Date | null | Yes | Left edge of the ellipse in units along the x axis. |
xScaleID | String - ScaleId | null | - | The ID of the X scale to bind onto. |
yMax | String - double - Date | null | Yes | Top edge of the ellipse in units along the y axis. |
yMin | String - double - Date | null | Yes | Bottom edge of the ellipse in units along the y axis. |
yScaleID | String - ScaleId | null | - | The ID of the Y scale to bind onto. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
Ellipse scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
EllipseAnnotation ellipse = new EllipseAnnotation();
// sets callback for border width options
ellipse.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(ellipse);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
adjustScaleRange | AdjustScaleRangeCallback | boolean |
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
drawTime | DrawTimeCallback | DrawTime |
rotation | RotationCallback<AnnotationContext> | double |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
xMax | ValueCallback | String - double - Date |
xMin | ValueCallback | String - double - Date |
yMax | ValueCallback | String - double - Date |
yMin | ValueCallback | String - double - Date |
z | ZCallback | int |
Ellipse label
An ellipse annotation can have a label to draw in the ellipse.
Every ellipse annotation can have ONLY 1 label.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
EllipseAnnotation ellipse = new EllipseAnnotation();
// sets label configuration
ellipse.getLabel().setDisplay(true);
ellipse.getLabel().setContent("My label");
// stores the annotation in the main options
options.setAnnotations(ellipse);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
color | String[] - IsColor[] | #fff - | Yes | The text color of the label. |
content | String - String[] - Img - Canvas | null | Yes | The content to show in the label. Provide an array to display values on a new line. |
display | boolean | false | Yes | Whether or not the label is shown. |
drawTime | DrawTime | See description | - | Defines when the label is drawn. Defaults to the box annotation draw time if unset. |
font | IsFont - List<FontItem> - FontItem[] | See description | Yes | The text font of the label. The default value is the global font with the weight set to Weight.BOLD. See Font. |
imageHeight | int - String | Undefined.INTEGER - null | Yes | Overrides the height of the image. Could be set in pixel by a number, or in percentage of current height of image by a string. If uset, uses the height of the image. It is used only when the content is an image. |
imageOpacity | double | 0 | Yes | Overrides the opacity of the image or canvas element. Could be set a number in the range 0.0 to 1.0, inclusive. It is used only when the content is an image or canvas element. |
imageWidth | int - String | Undefined.INTEGER - null | Yes | Overrides the width of the image. Could be set in pixel by a number, or in percentage of current width of image by a string. If unset, uses the width of the image. It is used only when the content is an image. |
padding | Padding | 6 | Yes | Number of pixels to add above and below the title text. See padding documentation for more details. |
position | AlignPosition | See position | Yes | Anchor position of label on box. |
rotation | double | 0 | Yes | The rotation of label, in degrees. |
textAlign | TextAlign | TextAlign.START | Yes | Horizontal alignment on the label content when is set as a multiple lines text. |
textStrokeColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The stroke color of the text. |
textStrokeWidth | int | 0 | Yes | The stroke width of the text. |
xAdjust | double | 0 | Yes | Adjustment along x-axis (left-right) of label relative to computed position. Negative values move the label left, positive right. |
yAdjust | double | 0 | Yes | Adjustment along y-axis (top-bottom) of label relative to computed position. Negative values move the label up, positive down. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
Ellipse label scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
EllipseAnnotation ellipse = new EllipseAnnotation();
// sets callback for color options
ellipse.getLabel().setColor(new ColorCallback<AnnotationContext>(){
@Override
public IsColor invoke(AnnotationContext context){
// logic
return color;
}
});
// stores the annotation in the main options
options.setAnnotations(ellipse);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
color | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
content | ContentCallback | String - List<String> - Img - Canvas |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
font | FontsCallback<AnnotationContext> | List<FontItem> |
imageHeight | ImageSizeCallback | String - int |
imageOpacity | ImageOpacityCallback | double |
imageWidth | ImageSizeCallback | String - int |
padding | PaddingCallback<AnnotationContext> | PaddingItem |
rotation | RotationCallback<AnnotationContext> | double |
position | LabelAlignPositionCallback | AlignPosition |
textAlign | TextAlignCallback<AnnotationContext> | TextAlign |
textStrokeColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
textStrokeWidth | WidthCallback<AnnotationContext> | int |
xAdjust | AdjustSizeCallback | double |
yAdjust | AdjustSizeCallback | double |
z | ZCallback | int |
Label
Label annotations are used to add contents on the chart area. This can be useful for describing values that are of interest.
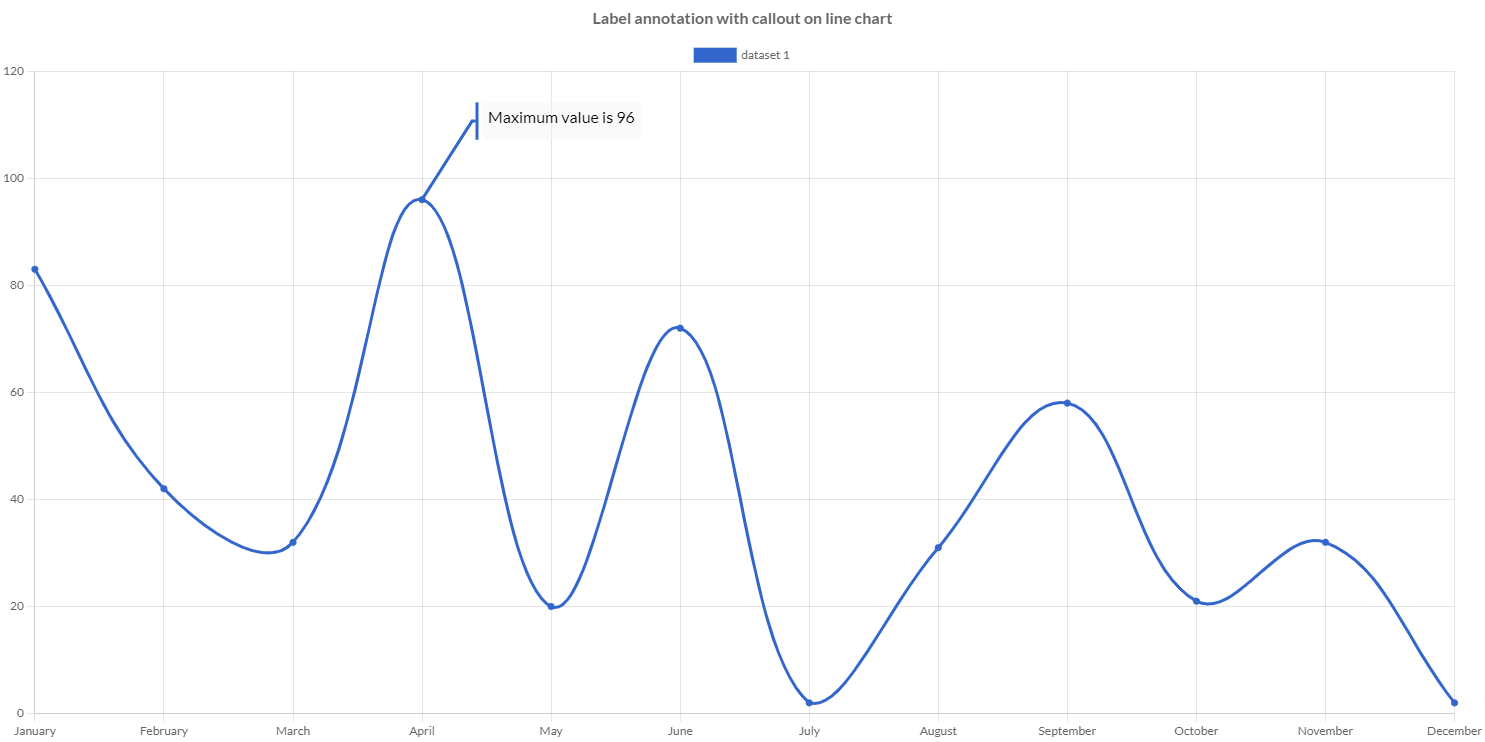
Every plugin options can have an unlimited number of labels annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
LabelAnnotation label = new LabelAnnotation();
label.setDrawTime(DrawTime.AFTER_DRAW);
label.setXScaleID(DefaultScaleId.X);
label.setYScaleID(DefaultScaleId.Y);
label.setXValue("February");
label.setYValue(50);
label.setContent("My label");
// stores the annotation in the main options
options.setAnnotations(label);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
adjustScaleRange | boolean | true | Yes | If true , the scale range should be adjusted if this annotation is out of range. |
backgroundColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The fill color of the label box. See default colors. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderCapStyle | CapStyle | CapStyle.BUTT | Yes | Cap style of the border label box. See MDN. |
borderColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The stroke color of the label box. See default colors. |
borderDash | int[] | [] | Yes | The length and spacing of dashes. See MDN. |
borderDashOffset | double | 0 | Yes | The offset for line dashes. See MDN. |
borderJoinStyle | JoinStyle | JoinStyle.MITER | Yes | Border line joint style. See MDN |
borderRadius | int - BarBorderRadius | 0 | Yes | The radius in pixels of label box. |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 0 | Yes | The stroke width of the label box. |
color | String[] - IsColor[] | HtmlColor.BLACK - | Yes | The text color of the label. |
content | String - String[] - Img - Canvas | null | Yes | The content to show in the label. Provide an array to display values on a new line. |
display | boolean | true | Yes | Whether or not this annotation is visible. |
drawTime | DrawTime | DrawTime. AFTER_DATASETS_DRAW | Yes | Defines when the annotation is drawn. This allows positioning of the annotation relative to the other elements of the graph. |
font | IsFont - List<FontItem> - FontItem[] | See description | Yes | The text font of the label. The default value is the global font. See Font. |
imageHeight | int - String | Undefined.INTEGER - null | Yes | Overrides the height of the image. Could be set in pixel by a number, or in percentage of current height of image by a string. If uset, uses the height of the image. It is used only when the content is an image. |
imageOpacity | double | 0 | Yes | Overrides the opacity of the image or canvas element. Could be set a number in the range 0.0 to 1.0, inclusive. It is used only when the content is an image or canvas element. |
imageWidth | int - String | Undefined.INTEGER - null | Yes | Overrides the width of the image. Could be set in pixel by a number, or in percentage of current width of image by a string. If unset, uses the width of the image. It is used only when the content is an image. |
init | boolean | false | - | If true or customized by callback, the annotation element will be animated since the initial phase. |
padding | Padding | 6 | Yes | Number of pixels to add above and below the title text. See padding documentation for more details. |
position | AlignPosition | See position | Yes | Anchor position of label. |
rotation | double | 0 | Yes | The rotation of annotation, in degrees. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
textAlign | TextAlign | TextAlign.CENTER | Yes | Horizontal alignment on the label content when is set as a multiple lines text. |
textStrokeColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The stroke color of the text. |
textStrokeWidth | int | 0 | Yes | The stroke width of the text. |
xAdjust | double | 0 | Yes | Adjustment along x-axis (left-right) of point relative to computed position. Negative values move the point left, positive right. |
xMax | String - double - Date | null | Yes | X coordinate of end two of the box, whose center is used as the center of the point, in units along the x axis. |
xMin | String - double - Date | null | Yes | X coordinate of end one of the box, whose center is used as the center of the point, in units along the x axis. |
xScaleID | String - ScaleId | null | - | The ID of the X scale to bind onto. |
xValue | String - double - Date | null | Yes | X coordinate of the center of point in units along the x axis. |
yAdjust | double | 0 | Yes | Adjustment along y-axis (top-bottom) of point relative to computed position. Negative values move the point up, positive down. |
yMax | String - double - Date | null | Yes | Y coordinate of end one of the box, whose center is used as the center of the point, in units along the y axis. |
yMin | String - double - Date | null | Yes | Y coordinate of end one of the box, whose center is used as the center of the point, in units along the y axis. |
yScaleID | String - ScaleId | null | - | The ID of the Y scale to bind onto. |
yValue | String - double - Date | null | Yes | Y coordinate of the center of point in units along the y axis. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
If one of the axes does not match an axis in the chart, the point annotation will take the center of the chart as point. The 2 coordinates, xValue, yValue are optional. If not specified, the point annotation will take the center of the chart as point.
The 4 coordinates, xMin, xMax, yMin, yMax are optional. If not specified, the box is expanded out to the edges in the respective direction and the box size is used to calculated the center of the point.
Label position
AlignPosition can define the x
property for the horizontal alignment of the label with respect to the selected point. Similarly, the y
property defines the vertical alignment.
Possible options for both properties are LabelPosition.START, LabelPosition.CENTER, LabelPosition.END, or a double, a value between 0 and 1, is representing the percentage on the size where the label will be located.
Default is LabelPosition.CENTER.
Label scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LabelAnnotation label = new LabelAnnotation();
// sets callback for border width options
label.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(label);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
adjustScaleRange | AdjustScaleRangeCallback | boolean |
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderCapStyle | CapStyleCallback<AnnotationContext> | CapStyle |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderJoinStyle | JoinStyleCallback<AnnotationContext> | JoinStyle |
borderRadius | BorderRadiusCallback<AnnotationContext> | int - - BarBorderRadius |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
color | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
content | ContentCallback | String - List<String> - Img - Canvas |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
drawTime | DrawTimeCallback | DrawTime |
font | FontsCallback<AnnotationContext> | List<FontItem> |
imageHeight | ImageSizeCallback | String - int |
imageOpacity | ImageOpacityCallback | double |
imageWidth | ImageSizeCallback | String - int |
padding | PaddingCallback<AnnotationContext> | PaddingItem |
position | LabelAlignPositionCallback | AlignPosition |
rotation | RotationCallback<AnnotationContext> | double |
textAlign | TextAlignCallback<AnnotationContext> | TextAlign |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
textStrokeColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
textStrokeWidth | WidthCallback<AnnotationContext> | int |
xAdjust | AdjustSizeCallback | double |
xMax | ValueCallback | String - double - Date |
xMin | ValueCallback | String - double - Date |
xValue | ValueCallback | String - double - Date |
yAdjust | AdjustSizeCallback | double |
yMax | ValueCallback | String - double - Date |
yMin | ValueCallback | String - double - Date |
yValue | ValueCallback | String - double - Date |
z | ZCallback | int |
Callout
The callout connects the label by a line to the selected point.
Every label annotation can have ONLY 1 callout.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
LabelAnnotation label = new LabelAnnotation();
// sets callout configuration
line.getCallout().setDisplay(true);
// stores the annotation in the main options
options.setAnnotations(line);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
borderCapStyle | CapStyle | CapStyle.BUTT | Yes | Cap style of the callout line. See MDN. |
borderColor | String - IsColor | HtmlColor.BLACK - | Yes | The color of the callout line. |
borderDash | int[] | [] | Yes | Length and spacing of dashes. See MDN. |
borderDashOffset | double | 0 | Yes | Offset for line dashes. See MDN. |
borderJoinStyle | JoinStyle | JoinStyle.MITER | Yes | Callout line joint style. See MDN. |
borderWidth | int | 1 | Yes | The width of the callout line in pixels. |
display | boolean | false | Yes | Whether or not the callout is shown. |
margin | int | 5 | Yes | Amount of pixels between the label and the callout separator. |
position | CalloutPosition | CalloutPosition.AUTO | Yes | The position of callout, with respect to the label. |
side | int | 5 | Yes | Width of the starter line of callout pointer. |
start | double - int | 0.5D | Yes | The percentage of the separator dimension to use as starting point for callout pointer. Could be set in pixel by an integer, or in percentage of the separator dimension by a double, a value between 0 and 1. |
Callout scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LabelAnnotation label = new LabelAnnotation();
// sets callback for border color options
label.getCallout().setBorderColor(new ColorCallback<AnnotationContext>(){
@Override
public IsColor invoke(AnnotationContext context){
// logic
return color;
}
});
// stores the annotation in the main options
options.setAnnotations(label);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
borderCapStyle | CapStyleCallback<AnnotationContext> | CapStyle |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderJoinStyle | JoinStyleCallback<AnnotationContext> | JoinStyle |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
margin | MarginCallback | int |
position | CalloutPositionCallback | CalloutPosition |
side | SideCallback | int |
start | [StartCallback](https://pepstock-org.github.io/Charba/6.5/org/pepstock/charba/client/annotation/callbacks/StartCallback](https.html) | double - int |
Line
Line annotations are used to draw lines on the chart area. This can be useful for highlighting information such as a threshold.
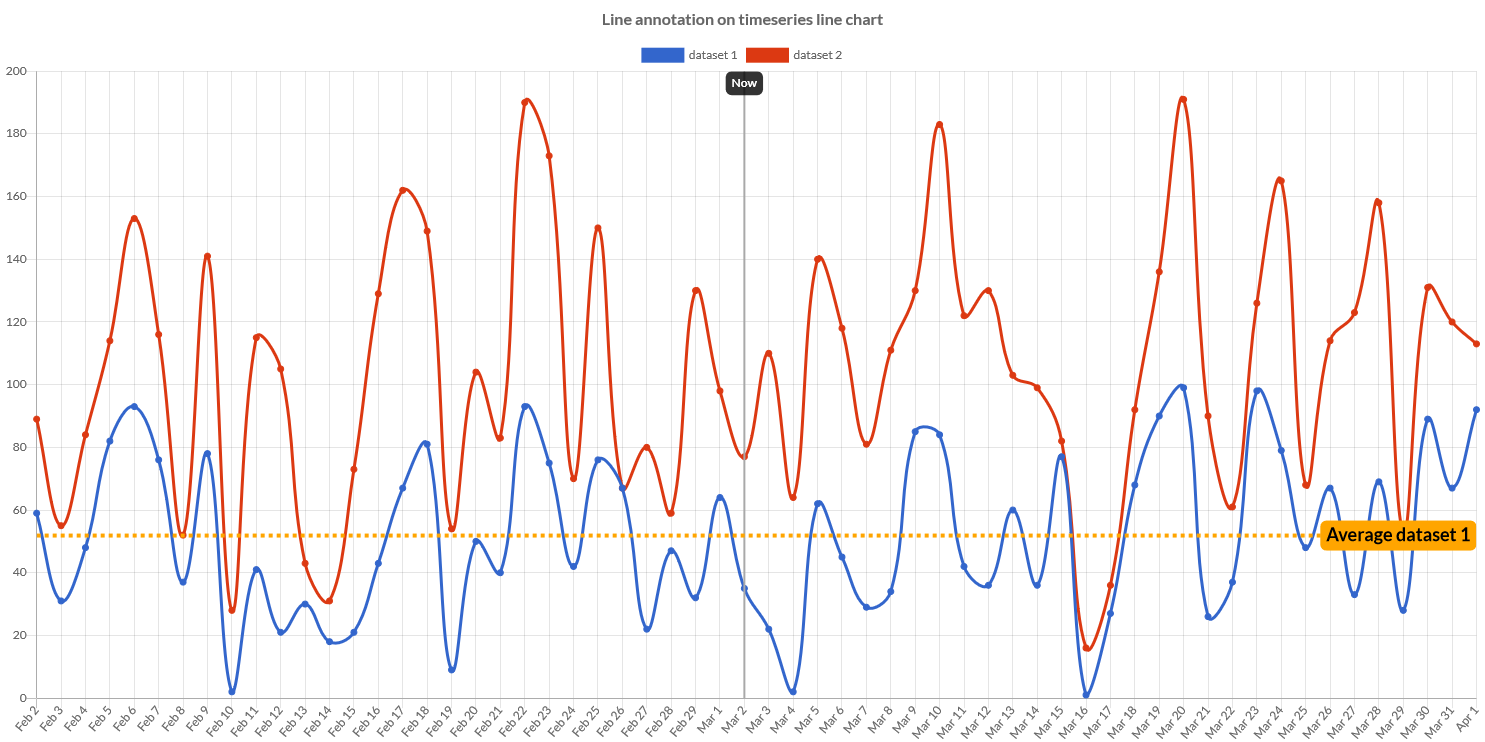
Every plugin options can have an unlimited number of lines annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
LineAnnotation line = new LineAnnotation();
line.setDrawTime(DrawTime.AFTER_DRAW);
line.setScaleID(DefaultScaleId.X.value());
line.setBorderColor(HtmlColor.DARK_GRAY);
line.setBorderWidth(2);
line.setValue(new Date());
// sets label configuration
line.getLabel().setDisplay(true);
line.getLabel().setContent("Now");
line.getLabel().setPosition(LabelPosition.START);
// stores the annotation in the main options
options.setAnnotations(line);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
adjustScaleRange | boolean | true | Yes | If true , the scale range should be adjusted if this annotation is out of range. |
borderColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The stroke color of the line. |
borderDash | int[] | [] | Yes | the line dash pattern used when stroking lines, using an array of values which specify alternating lengths of lines and gaps which describe the pattern. |
borderDashOffset | int | 0 | Yes | Offset for line dashes. See MDN |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 2 | Yes | The stroke width of the line. |
controlPoint | double - String - ControlPoint | {y:'-50%'} | Yes | If curve is enabled, it configures the control point to drawn the curve, calculated in pixels. It can be set by a string in percentage format "number%" which are representing the percentage of the distance between the start and end point from the center. |
curve | boolean | false | Yes | Whether or not a quadratic Bézier curve is drawn. |
display | boolean | true | Yes | Whether or not this annotation is visible. |
drawTime | DrawTime | DrawTime. AFTER_DATASETS_DRAW | Yes | Defines when the annotation is drawn. This allows positioning of the annotation relative to the other elements of the graph. |
endValue | String - double - Date | null | Yes | End two of the line when a single scale is specified. |
init | boolean | false | - | If true or customized by callback, the annotation element will be animated since the initial phase. |
scaleID | String - ScaleId | null | - | ID of the scale in single scale mode. If unset, xScaleID and yScaleID are used. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
value | String - double - Date | null | Yes | End one of the line when a single scale is specified. |
xMax | String - double - Date | null | Yes | X coordinate of end two of the line in units along the x axis. |
xMin | String - double - Date | null | Yes | X coordinate of end one of the line in units along the x axis. |
xScaleID | String - ScaleId | null | - | The ID of the X scale to bind onto. |
yMax | String - double - Date | null | Yes | Y coordinate of end one of the line in units along the y axis. |
yMin | String - double - Date | null | Yes | Y coordinate of end one of the line in units along the y axis. |
yScaleID | String - ScaleId | null | - | The ID of the Y scale to bind onto. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
If one of the axes does not match an axis in the chart, the line will take the entire chart dimension.
The 4 coordinates, xMin, xMax, yMin, yMax are optional. If not specified, the line is expanded out to the edges in the respective direction.
The line can be positioned in two different ways:
- if
scaleID
is set, thenvalue
andendValue
must also be set to indicate the end points of the line. The line will be perpendicular to the axis identified byscaleID
. - if
scaleID
is unset, thenxScaleID
andyScaleID
are used to draw a line from(xMin, yMin)
to(xMax, yMax)
.
Line scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LineAnnotation line = new LineAnnotation();
// sets callback for border width options
line.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(line);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
adjustScaleRange | AdjustScaleRangeCallback | boolean |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
controlPoint | ControlPointCallback | double - String - ControlPoint |
curve | CurveCallback | boolean |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
drawTime | DrawTimeCallback | DrawTime |
endValue | ValueCallback | String - double - Date |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
value | ValueCallback | String - double - Date |
xMax | ValueCallback | String - double - Date |
xMin | ValueCallback | String - double - Date |
yMax | ValueCallback | String - double - Date |
yMin | ValueCallback | String - double - Date |
z | ZCallback | int |
Line label
A line annotation can have a label to draw on the line.
Every line annotation can have ONLY 1 label.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
LineAnnotation line = new LineAnnotation();
// sets label configuration
line.getLabel().setDisplay(true);
line.getLabel().setContent("My threshold");
line.getLabel().setBackgroundColor(HtmlColor.RED);
// stores the annotation in the main options
options.setAnnotations(line);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
autoRotation | boolean | false | - | If true , the rotation option is ignored and the label uses the degrees of the line. |
backgroundColor | String - IsColor | rgba(0,0,0,0.8) - | Yes | Background color of the label container. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderCapStyle | CapStyle | CapStyle.BUTT | Yes | Cap style of the line. See MDN. |
borderColor | String - IsColor | HtmlColor.BLACK - | Yes | The color of the line. |
borderDash | int[] | [] | Yes | Length and spacing of dashes. See MDN. |
borderDashOffset | double | 0 | Yes | Offset for line dashes. See MDN. |
borderJoinStyle | JoinStyle | JoinStyle.MITER | Yes | Line joint style. See MDN. |
borderRadius | int - BarBorderRadius | 6 | Yes | The radius of label box in pixels. |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 0 | Yes | The width of the line in pixels. |
color | String[] - IsColor[] | #fff - | Yes | The text color of the label. |
content | String - String[] - Img - Canvas | null | Yes | The content to show in the label. Provide an array to display values on a new line. |
display | boolean | false | Yes | Whether or not the label is shown. |
drawTime | DrawTime | See description | - | Defines when the label is drawn. Defaults to the line annotation draw time if unset. |
font | IsFont - List<FontItem> - FontItem[] | See description | Yes | The text font of the label. The default value is the global font with the style set to FontStyle.BOLD. See Font. |
imageHeight | int - String | Undefined.INTEGER - null | Yes | Overrides the height of the image. Could be set in pixel by a number, or in percentage of current height of image by a string. If uset, uses the height of the image. It is used only when the content is an image. |
imageOpacity | double | 0 | Yes | Overrides the opacity of the image or canvas element. Could be set a number in the range 0.0 to 1.0, inclusive. It is used only when the content is an image or canvas element. |
imageWidth | int - String | Undefined.INTEGER - null | Yes | Overrides the width of the image. Could be set in pixel by a number, or in percentage of current width of image by a string. If unset, uses the width of the image. It is used only when the content is an image. |
padding | Padding | 6 | Yes | Number of pixels to add above and below the title text. See padding documentation for more details. |
position | LabelPosition - double | LabelPosition.CENTER | Yes | Anchor position of label on line. If set by a double, a value between 0 and 1, is representing the percentage on the size where the label will be located. |
rotation | double | 0 | Yes | The rotation of label, in degrees. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
textAlign | TextAlign | TextAlign.CENTER | Yes | Horizontal alignment on the label content when is set as a multiple lines text. |
textStrokeColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The stroke color of the text. |
textStrokeWidth | int | 0 | Yes | The stroke width of the text. |
xAdjust | double | 0 | Yes | Adjustment along x-axis (left-right) of label relative to computed position. Negative values move the label left, positive right. |
yAdjust | double | 0 | Yes | Adjustment along y-axis (top-bottom) of label relative to computed position. Negative values move the label up, positive down. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
Line label scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LineAnnotation line = new LineAnnotation();
// sets callback for background color options
line.getLabel().setBackgroundColor(new ColorCallback<AnnotationContext>(){
@Override
public IsColor invoke(AnnotationContext context){
// logic
return color;
}
});
// stores the annotation in the main options
options.setAnnotations(line);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderCapStyle | CapStyleCallback<AnnotationContext> | CapStyle |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderJoinStyle | JoinStyleCallback<AnnotationContext> | JoinStyle |
borderRadius | BorderRadiusCallback<AnnotationContext> | int - - BarBorderRadius |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
color | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
content | ContentCallback | String - List<String> - Img - Canvas |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
font | FontsCallback<AnnotationContext> | List<FontItem> |
imageHeight | ImageSizeCallback | String - int |
imageOpacity | ImageOpacityCallback | double |
imageWidth | ImageSizeCallback | String - int |
padding | PaddingCallback<AnnotationContext> | PaddingItem |
position | LabelPositionCallback | double - LabelPosition |
rotation | RotationCallback<AnnotationContext> | double(1) |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
textAlign | TextAlignCallback<AnnotationContext> | TextAlign |
textStrokeColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
textStrokeWidth | WidthCallback<AnnotationContext> | int |
xAdjust | AdjustSizeCallback | double |
yAdjust | AdjustSizeCallback | double |
z | ZCallback | int |
(1)To enable autoRotation
by the rotation callback, the value to return must be Double.NaN
.
// sets callback for auto rotation
line.getLabel().setRotation(new RotationCallback<AnnotationContext>(){
@Override
public Double invoke(AnnotationContext context){
return Double.NaN; // autoRotation is set
}
});
Callout
The callout connects the label by a line to the line annotation.
Every line annotation can have ONLY 1 line label and callout.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
LineAnnotation line = new LineAnnotation();
// sets label configuration
line.getLabel().setDisplay(true);
line.getLabel().setContent("My threshold");
line.getLabel().setBackgroundColor(HtmlColor.RED);
line.getLabel().setYAdjust(-100);
// sets callout configuration
line.getLabel().getCallout().setDisplay(true);
// stores the annotation in the main options
options.setAnnotations(line);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
borderCapStyle | CapStyle | CapStyle.BUTT | Yes | Cap style of the callout line. See MDN. |
borderColor | String - IsColor | HtmlColor.BLACK - | Yes | The color of the callout line. |
borderDash | int[] | [] | Yes | Length and spacing of dashes. See MDN. |
borderDashOffset | double | 0 | Yes | Offset for line dashes. See MDN. |
borderJoinStyle | JoinStyle | JoinStyle.MITER | Yes | Callout line joint style. See MDN. |
borderWidth | int | 1 | Yes | The width of the callout line in pixels. |
display | boolean | false | Yes | Whether or not the callout is shown. |
margin | int | 5 | Yes | Amount of pixels between the label and the callout separator. |
position | CalloutPosition | CalloutPosition.AUTO | Yes | The position of callout, with respect to the label. |
side | int | 5 | Yes | Width of the starter line of callout pointer. |
start | double - int | 0.5D | Yes | The percentage of the separator dimension to use as starting point for callout pointer. Could be set in pixel by an integer, or in percentage of the separator dimension by a double, a value between 0 and 1. |
Line label Callout scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LineAnnotation line = new LineAnnotation();
// sets label configuration
line.getLabel().setDisplay(true);
line.getLabel().setContent("My threshold");
line.getLabel().setBackgroundColor(HtmlColor.RED);
line.getLabel().setYAdjust(-100);
line.getLabel().getCallout().setDisplay(true);
// sets callback for border color options
line.getLabel().getCallout().setBorderColor(new ColorCallback<AnnotationContext>(){
@Override
public IsColor invoke(AnnotationContext context){
// logic
return color;
}
});
// stores the annotation in the main options
options.setAnnotations(label);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
borderCapStyle | CapStyleCallback<AnnotationContext> | CapStyle |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderJoinStyle | JoinStyleCallback<AnnotationContext> | JoinStyle |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
margin | MarginCallback | int |
position | CalloutPositionCallback | CalloutPosition |
side | SideCallback | int |
start | [StartCallback](https://pepstock-org.github.io/Charba/6.5/org/pepstock/charba/client/annotation/callbacks/StartCallback](https.html) | double - int |
Arrow heads
Enabling it, you can add arrow heads at start and/or end of a line. It uses the borderWidth
of the line options to configure the line width of the arrow head.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
LineAnnotation line = new LineAnnotation();
// sets arrowheads configuration
line.getArrowHeads().setDisplay(true);
line.getArrowHeads().setLength(20);
line.getArrowHeads().setWidth(12);
// stores the annotation in the main options
options.setAnnotations(line);
The following options can be specified per (line.getArrowHeads().getStart()
and/or line.getArrowHeads().getEnd()
) arrow head, or at the top level (line.getArrowHeads()
) which apply to all arrow heads.
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
backgroundColor | String - IsColor | lineAnnotation.borderColor | Yes | Background color of the arrow head. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderColor | String - IsColor | lineAnnotation.borderColor | Yes | The border arrow head color. |
borderDash | int[] | lineAnnotation.borderDash | Yes | Length and spacing of dashes. See MDN. |
borderDashOffset | double | lineAnnotation.borderDashOffset | Yes | Offset for line dashes. See MDN. |
borderShadowColor | String - IsColor | lineAnnotation.borderShadowColor | Yes | The color of border shadows. See MDN. |
borderWidth | int | lineAnnotation.borderWidth | Yes | The width of the line in pixels. |
display | boolean | false | Yes | Whether or not the arrow head is shown. |
end | ArrowHeads | - | - | To configure the arrow head at the end of the line. |
fill | boolean | false | Yes | Whether or not the arrow head is filled. |
length | int | 12 | Yes | The length of the arrow head in pixels. |
shadowBlur | double | lineAnnotation.shadowBlur | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | lineAnnotation.shadowOffsetX | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | lineAnnotation.shadowOffsetY | Yes | The distance that shadows will be offset vertically. See MDN. |
start | ArrowHeads | - | - | To configure the arrow head at the start of the line. |
width | int | 6 | Yes | The width of the arrow head in pixels. |
ArrowHeads scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
LineAnnotation line = new LineAnnotation();
// sets callback for background color options
line.getArrowHeads().setBackgroundColor(new ColorCallback<AnnotationContext>(){
@Override
public IsColor invoke(AnnotationContext context){
// logic
return color;
}
});
// stores the annotation in the main options
options.setAnnotations(line);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
fill | FillCallback | boolean |
length | LengthCallback | int |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
width | WidthCallback<AnnotationContext> | int |
Point
Point annotations are used to mark points on the chart area. This can be useful for highlighting values that are of interest.
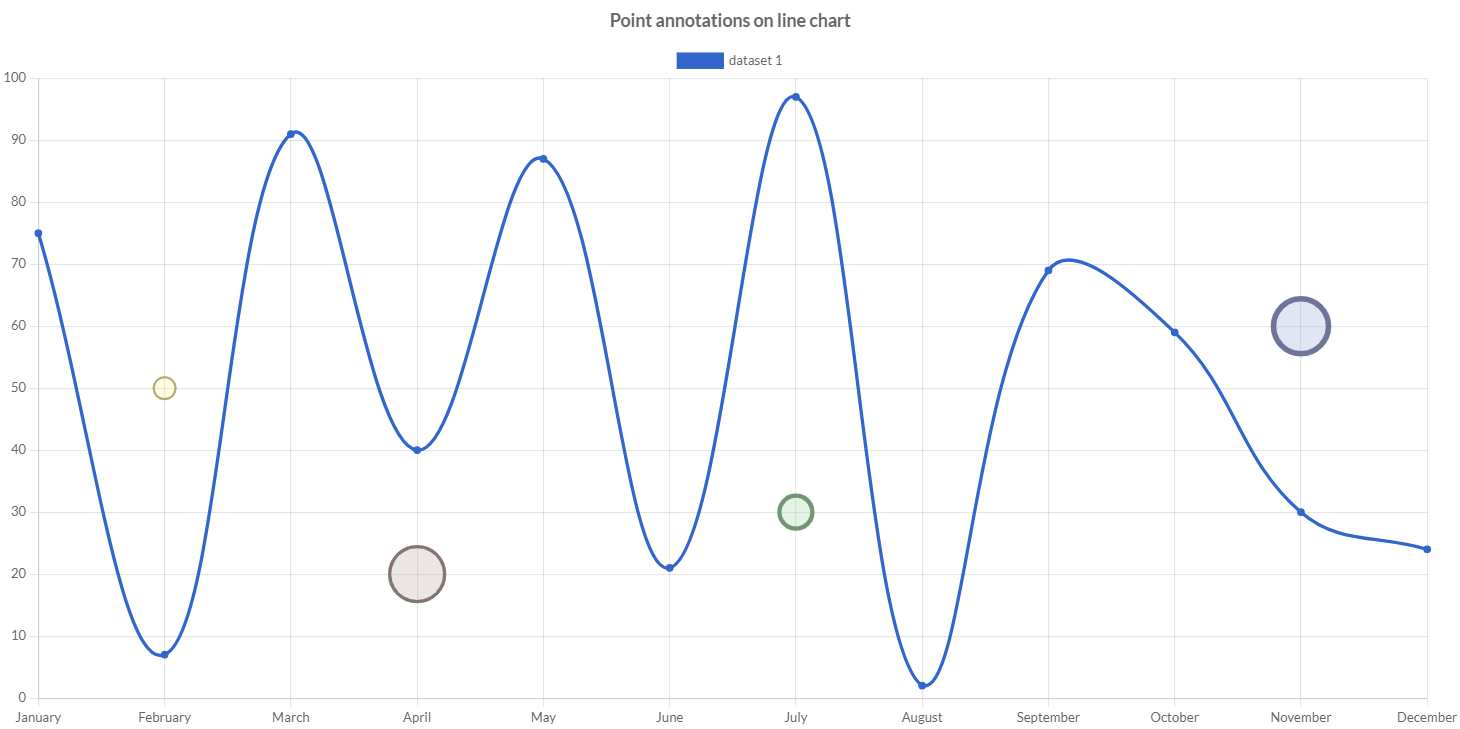
Every plugin options can have an unlimited number of points annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
PointAnnotation point = new PointAnnotation();
// sets annotation configuration
point.setXScaleID(DefaultScaleId.X);
point.setYScaleID(DefaultScaleId.Y);
point.setXValue("February");
point.setYValue(50);
point.setRadius(10);
point.setBackgroundColor(HtmlColor.YELLOW.alpha(0.3D));
point.setBorderWidth(2);
point.setBorderColor(HtmlColor.YELLOW.darker());
// stores the annotation in the main options
options.setAnnotations(point);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
adjustScaleRange | boolean | true | Yes | If true , the scale range should be adjusted if this annotation is out of range. |
backgroundColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The fill color of the point. See default colors. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The stroke color of the point. |
borderDash | int[] | [] | Yes | The line dash pattern used when stroking lines, using an array of values which specify alternating lengths of lines and gaps which describe the pattern. |
borderDashOffset | int | 0 | Yes | Offset for border dashes. See MDN |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 1 | Yes | The stroke width of the point. |
display | boolean | true | Yes | Whether or not this annotation is visible. |
drawTime | DrawTime | DrawTime. AFTER_DATASETS_DRAW | Yes | Defines when the annotation is drawn. This allows positioning of the annotation relative to the other elements of the graph. |
init | boolean | false | - | If true or customized by callback, the annotation element will be animated since the initial phase. |
pointStyle | PointStyle - Img - Canvas | PointStyle.CIRCLE | Yes | Style of the point. |
radius | double | 10 | Yes | Size of the point in pixels. |
rotation | double | 0 | Yes | The rotation of the point, in degrees. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
xAdjust | double | 0 | Yes | Adjustment along x-axis (left-right) of point relative to computed position. Negative values move the point left, positive right. |
xMax | String - double - Date | null | Yes | X coordinate of end two of the box, whose center is used as the center of the point, in units along the x axis. |
xMin | String - double - Date | null | Yes | X coordinate of end one of the box, whose center is used as the center of the point, in units along the x axis. |
xScaleID | String - ScaleId | null | - | The ID of the X scale to bind onto. |
xValue | String - double - Date | null | Yes | X coordinate of the center of point in units along the x axis. |
yAdjust | double | 0 | Yes | Adjustment along y-axis (top-bottom) of point relative to computed position. Negative values move the point up, positive down. |
yMax | String - double - Date | null | Yes | Y coordinate of end one of the box, whose center is used as the center of the point, in units along the y axis. |
yMin | String - double - Date | null | Yes | Y coordinate of end one of the box, whose center is used as the center of the point, in units along the y axis. |
yScaleID | String - ScaleId | null | - | The ID of the Y scale to bind onto. |
yValue | String - double - Date | null | Yes | Y coordinate of the center of point in units along the y axis. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
If one of the axes does not match an axis in the chart, the point annotation will take the center of the chart as point. The 2 coordinates, xValue, yValue are optional. If not specified, the point annotation will take the center of the chart as point.
The 4 coordinates, xMin, xMax, yMin, yMax are optional. If not specified, the box is expanded out to the edges in the respective direction and the box size is used to calculated the center of the point. To enable to use the box positioning, the radius
must be set to 0
.
Point scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
PointAnnotation point = new PointAnnotation();
// sets callback for border width options
point.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(point);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
adjustScaleRange | AdjustScaleRangeCallback | boolean |
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
drawTime | DrawTimeCallback | DrawTime |
pointStyle | PointStyleCallback<AnnotationContext> | PointStyle - Img - Canvas |
radius | RadiusCallback<AnnotationContext> | double |
rotation | RotationCallback<AnnotationContext> | double |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
xAdjust | AdjustSizeCallback | double |
xMax | ValueCallback | String - double - Date |
xMin | ValueCallback | String - double - Date |
xValue | ValueCallback | String - double - Date |
yAdjust | AdjustSizeCallback | double |
yMax | ValueCallback | String - double - Date |
yMin | ValueCallback | String - double - Date |
yValue | ValueCallback | String - double - Date |
z | ZCallback | int |
Polygon
Polygon annotations are used to mark whatever polygon (for instance triangle, square or pentagon) on the chart area. This can be useful for highlighting values that are of interest.
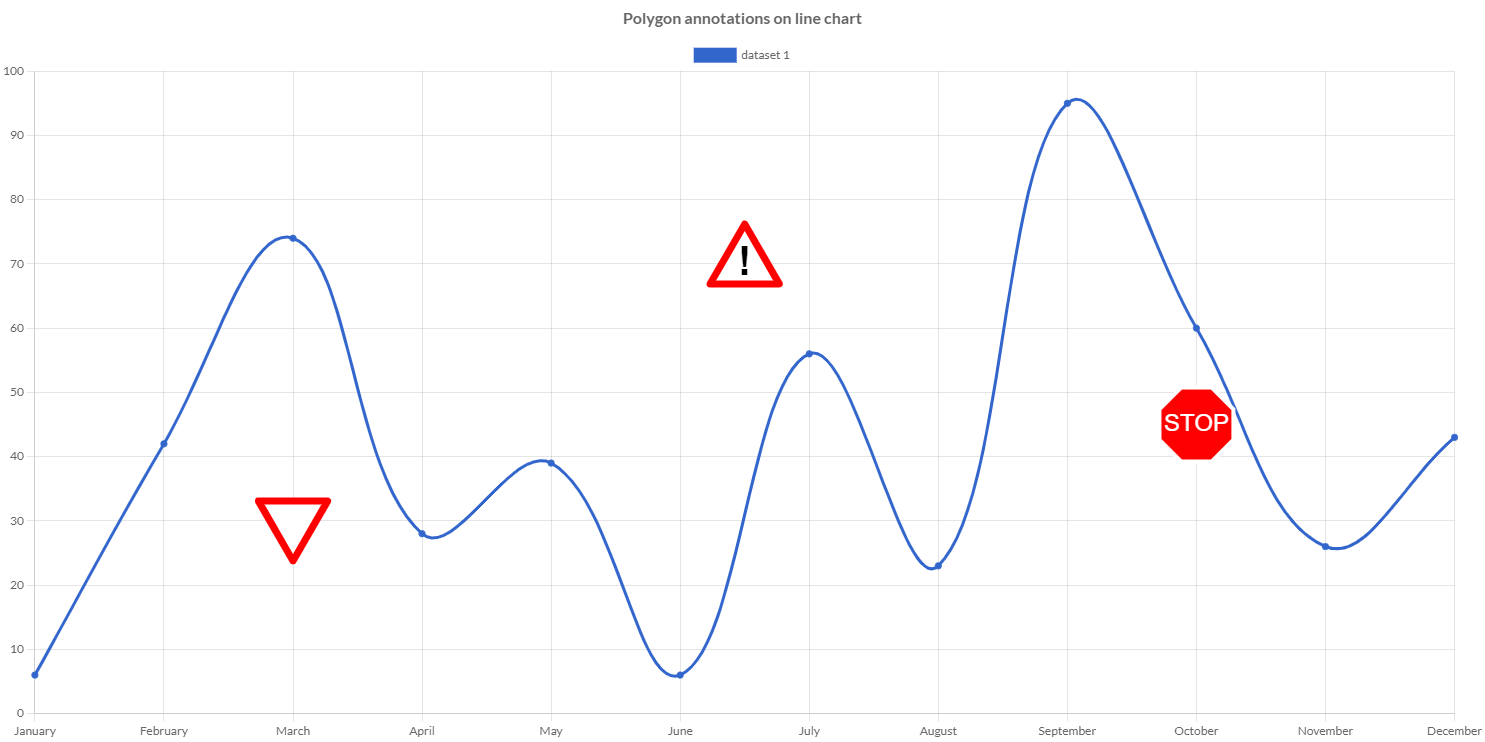
Every plugin options can have an unlimited number of polygons annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
PolygonAnnotation polygon = new PolygonAnnotation();
// sets annotation configuration
polygon.setXScaleID(DefaultScaleId.X);
polygon.setYScaleID(DefaultScaleId.Y);
polygon.setXValue("February");
polygon.setYValue(50);
polygon.setRadius(10);
// pentagon
polygon.setSides(5);
polygon.setBackgroundColor(HtmlColor.YELLOW.alpha(0.3D));
polygon.setBorderWidth(2);
polygon.setBorderColor(HtmlColor.YELLOW.darker());
// stores the annotation in the main options
options.setAnnotations(polygon);
The complete options are described by following table:
Name | Type | Default | Scriptable | Description |
---|---|---|---|---|
adjustScaleRange | boolean | true | Yes | If true , the scale range should be adjusted if this annotation is out of range. |
backgroundColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The fill color of the polygon. See default colors. |
backgroundShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of shadows. See MDN. |
borderCapStyle | CapStyle | CapStyle.BUTT | Yes | Cap style of the border line. See MDN. |
borderColor | String - IsColor | Defaults.get().getGlobal() .getColorAsString() | Yes | The stroke color of the polygon. |
borderDash | int[] | [] | Yes | The line dash pattern used when stroking lines, using an array of values which specify alternating lengths of lines and gaps which describe the pattern. |
borderDashOffset | int | 0 | Yes | Offset for border dashes. See MDN |
borderJoinStyle | JoinStyle | JoinStyle.MITER | Yes | Border line joint style. See MDN |
borderShadowColor | String - IsColor | HtmlColor.TRANSPARENT | Yes | The color of border shadows. See MDN. |
borderWidth | int | 1 | Yes | The stroke width of the polygon. |
display | boolean | true | Yes | Whether or not this annotation is visible. |
drawTime | DrawTime | DrawTime. AFTER_DATASETS_DRAW | Yes | Defines when the annotation is drawn. This allows positioning of the annotation relative to the other elements of the graph. |
init | boolean | false | - | If true or customized by callback, the annotation element will be animated since the initial phase. |
radius | double | 10 | Yes | Size of the polygon in pixels. |
rotation | double | 0 | Yes | The rotation of the polygon, in degrees. |
shadowBlur | double | 0 | Yes | The amount of blur applied to shadows. See MDN. |
shadowOffsetX | int | 0 | Yes | The distance that shadows will be offset horizontally. See MDN. |
shadowOffsetY | int | 0 | Yes | The distance that shadows will be offset vertically. See MDN. |
sides | int | 3 | Yes | Amount of sides of polygon. |
xAdjust | double | 0 | Yes | Adjustment along x-axis (left-right) of polygon relative to computed position. Negative values move the polygon left, positive right. |
xMax | String - double - Date | null | Yes | X coordinate of end two of the box, whose center is used as the center of the polygon, in units along the x axis. |
xMin | String - double - Date | null | Yes | X coordinate of end one of the box, whose center is used as the center of the polygon, in units along the x axis. |
xScaleID | String - ScaleId | null | - | The ID of the X scale to bind onto. |
xValue | String - double - Date | null | Yes | X coordinate of the center of polygon in units along the x axis. |
yAdjust | double | 0 | Yes | Adjustment along y-axis (top-bottom) of polygon relative to computed position. Negative values move the polygon up, positive down. |
yMax | String - double - Date | null | Yes | Y coordinate of end one of the box, whose center is used as the center of the polygon, in units along the y axis. |
yMin | String - double - Date | null | Yes | Y coordinate of end one of the box, whose center is used as the center of the polygon, in units along the y axis. |
yScaleID | String - ScaleId | null | - | The ID of the Y scale to bind onto. |
yValue | String - double - Date | null | Yes | Y coordinate of the center of polygon in units along the y axis. |
z | int | 0 | Yes | All visible elements will be drawn in ascending order of z option, with the same drawTime option. |
If one of the axes does not match an axis in the chart, the point annotation will take the center of the chart as point. The 2 coordinates, xValue, yValue are optional. If not specified, the point annotation will take the center of the chart as point.
The 4 coordinates, xMin, xMax, yMin, yMax are optional. If not specified, the box is expanded out to the edges in the respective direction and the box size is used to calculated the center of the point. To enable to use the box positioning, the radius
must be set to 0
.
Polygon scriptable options
Some options also accept a callback which is called at runtime and that takes the context as single argument, see here the details, which is representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
PolygonAnnotation polygon = new PolygonAnnotation();
// sets callback for border width options
polygon.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(polygon);
The following options can be set by a callback:
Name | Callback | Returned types |
---|---|---|
adjustScaleRange | AdjustScaleRangeCallback | boolean |
backgroundColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> - Pattern |
backgroundShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderCapStyle | CapStyleCallback<AnnotationContext> | CapStyle |
borderColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderDash | BorderDashCallback<AnnotationContext> | List<Integer> |
borderDashOffset | BorderDashOffsetCallback<AnnotationContext> | double |
borderJoinStyle | JoinStyleCallback<AnnotationContext> | JoinStyle |
borderShadowColor | ColorsCallback<AnnotationContext> | List<String> - List<IsColor> |
borderWidth | WidthCallback<AnnotationContext> | int |
display | SimpleDisplayCallback<AnnotationContext> | boolean |
drawTime | DrawTimeCallback | DrawTime |
radius | RadiusCallback<AnnotationContext> | double |
rotation | RotationCallback<AnnotationContext> | double |
shadowBlur | ShadowBlurCallback | double |
shadowOffsetX | ShadowOffsetCallback | int |
shadowOffsetY | ShadowOffsetCallback | int |
sides | SidesCallback | int |
xAdjust | AdjustSizeCallback | double |
xMax | ValueCallback | String - double - Date |
xMin | ValueCallback | String - double - Date |
xValue | ValueCallback | String - double - Date |
yAdjust | AdjustSizeCallback | double |
yMax | ValueCallback | String - double - Date |
yMin | ValueCallback | String - double - Date |
yValue | ValueCallback | String - double - Date |
z | ZCallback | int |
Events
All annotations provide a set of callbacks which can be enabled to catch events on them.
To catch events is enough to set the events which you want to catch at AnnotationOptions and set a callback instance in the annotation.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
// without id (a unique one is created automatically)
BoxAnnotation box = new BoxAnnotation();
// sets callback for specific annotation
box.setClickCallback(new ClickCallback(){
@Override
public boolean onClick(IsChart chart, AbstractAnnotation annotation, ChartEventContext event){
// logic
return false; // no redrawing
}
});
You can also catch events with listeners defined for all defined annotations with a single instance, defining it to AnnotationOptions.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// sets callback for all annotations
options.setClickCallback(new ClickCallback(){
@Override
public boolean onClick(IsChart chart, AbstractAnnotation annotation, ChartEventContext event){
// logic
return false; // no redrawing
}
});
These are the table of callbacks to implement:
Event | Callback type | Description |
---|---|---|
enter | EnterCallback | Called when the mouse enters the annotation. |
leave | LeaveCallback | Called when the mouse leaves the annotation. |
click | ClickCallback | Called when a click occurs on the annotation. |
If the event callback returns true
, the chart will re-render automatically after processing the event completely. This is important when there are the annotations that require re-draws (for instance, after a change of a rendering options).
Hooks
All annotations provide 2 hooks which can be enabled to be notified before and after the annotation drawing.
These hooks enable the user to apply own custom shapes or drawings on top to the existing annotations.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
BoxAnnotation box = new BoxAnnotation();
// sets callback
box.setBeforeDraw(new ElementHookCallback(){
@Override
public void invoke(AnnotationContext context){
// logic
}
});
// sets callback
box.setAfterDraw(new ElementHookCallback(){
@Override
public void invoke(AnnotationContext context){
// logic
}
});
Hook | Callback type | Description |
---|---|---|
beforeDraw | ElementHookCallback | Called before that the annotation is being drawn. |
afterDraw | ElementHookCallback | Called after the annotation has been drawn. |
Scriptable context
Some options also accept a callback which is called at runtime and that takes the context as single argument, AnnotationContext representing contextual information and chart instance.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
BoxAnnotation box = new BoxAnnotation();
// sets callback for border width options
box.setBorderWidth(new WidthCallback<AnnotationContext>(){
@Override
public Integer invoke(AnnotationContext context){
// logic
return borderWidth;
}
});
// stores the annotation in the main options
options.setAnnotations(box);
The context object contains the following properties:
Name | Type | Description |
---|---|---|
annotation | AbstractAnnotation | The annotation configuration where the options is defined as scriptable. |
attributes | NativeObjectContainer | User object which you can store your options at runtime. |
chart | IsChart | Chart instance. |
element | AnnotationElement | The element instance related to the annotation. |
shared | Map<String, Object> | A map that can be used to share objects among all contexts for all annotation. |
type | ContextType | The type of the context. It can be ContextType.CHART or ContextType.ANNOTATION |
Fonts and colors
When the label to draw has multiple lines, you can use different font and color for each row of the label. This is enabled configuring an array or list of fonts or colors for those options. When the lines are more than the configured fonts of colors, the last configuration of those options is used for all remaining lines.
Initial animation
The init
option is scriptable but it doesn't get the annotation context as argument but a specific context because the element has not been initialized yet, when the callback is invoked.
You can implement a InitCallback interface to enable your own initial animation of the annotation.
// creates a plugin options
AnnotationOptions options = new AnnotationOptions();
// creates an annotation
BoxAnnotation box = new BoxAnnotation();
// sets callback
box.setInit(new InitCallback(){
/**
* Invoked to enable a custom logic to apply the animation initialization.
*
* @param chart chart instance
* @param properties initializing annotation element properties
* @param options annotation options
* @return could be a boolean or an AnnotationProperties
*/
@Override
public Object invoke(IsChart chart, AnnotationProperties properties, AbstractAnnotation options){
AnnotationProperties initAnimProps = new AnnotationProperties();
// logic
return initAnimProps;
}
});
// stores the annotation in the main options
options.setAnnotations(box);