Geographic map charts
Charba provides out of the box the feature to enable some geographic map chart, leveraging on Chart.js Geo.
The Chart.js Geo is native javascript implementation and Charba provides the wrapper in order to be able to use it.
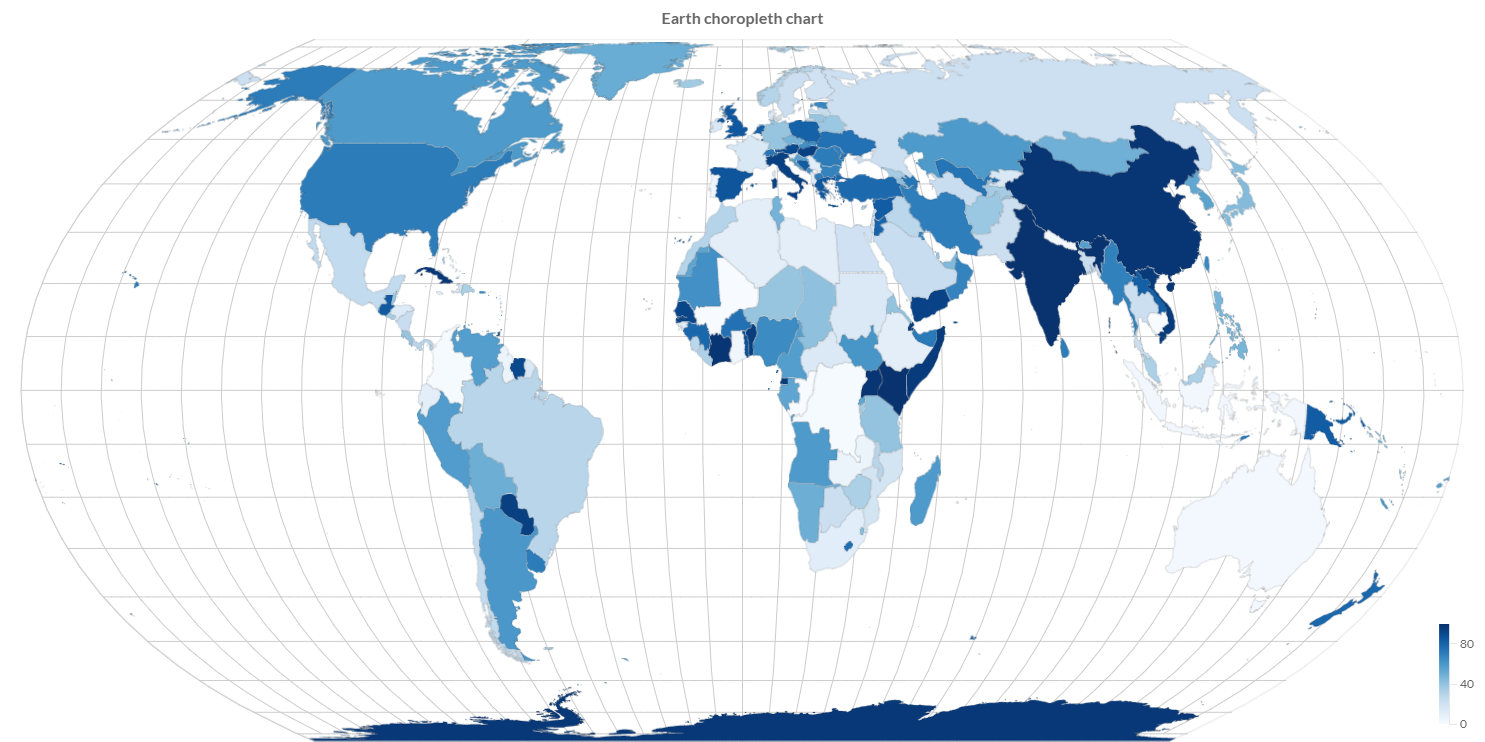
Currently the Chart.js Geo, ready to use inside Charba, can provide the following charts types:
- Choropleth which can be used to render maps with the area filled according to some numerical value.
- BubbleMap, as know as Proportional Symbol, which is used to render maps with dots that are scaled according to some numerical value.
Maps
The maps used to render the chart must be based on TopoJSON definitions.
TopoJSON is an extension of GeoJSON that encodes topology. TopoJSON is a topological geospatial data format. In contrast to a geometry, where each shape is encoded with separate (and often redundant) arrays of coordinates, a topology encodes sequences of coordinates in line fragments called arcs that can be shared. For example, the border between 2 states is an arc that is shared by both polygons.
The main benefit of a topology is that it improves shape simplification by avoiding artifacts that would be caused by simplifying shapes independently. It also enables applications like map coloring and selective meshing, and makes the format more compact by removing redundant coordinates.
Here is the TopoJSON specification
However, Charba does not include any TopoJSON files itself. Some useful resources are the following:
- US map: https://www.npmjs.com/package/us-atlas
- World map: https://www.npmjs.com/package/world-atlas
- TopoJSON collection: https://github.com/deldersveld/topojson
Loading
The maps can be loaded:
- at runtime, invoking some HTTP services in order to get the needed TopoJSON definitions.
- at project level, by GWT Web toolkit clientBundle, if you are developing a GWT Web toolkit project.
- at project level, by the implementation of a AbstractInjectableResource class.
An example how to load a map by GWT Web toolkit clientBundle is the following:
- take the TopoJSON definition and store in your project in a resource folder.
- create a GWT ClientBundle to get the TopoJSON definition as GWT TextResource
- use GeoUtil to get a TopoJson instance.
An example how to load a map by GWT Web toolkit clientBundle is the following:
- get the world map JSON file from NPMjs and store it in your project, in this example we are using the Charba showcase paths:
/src/org/pepstock/charba/showcase/client/resources/topojson/countries-50m.json
- create a client bundle (called in this example
org.pepstock.charba.showcase.client.resources.MyResources.java
) with the TopoJSON map reference:
package org.pepstock.charba.showcase.client.resources;
import com.google.gwt.core.client.GWT;
import com.google.gwt.resources.client.ClientBundle;
import com.google.gwt.resources.client.TextResource;
public interface MyResources extends ClientBundle {
public static final MyResources INSTANCE = GWT.create(MyResources.class);
@Source("topojson/countries-50m.json")
TextResource topojsonEarth();
}
// gets the topoJSON map as string
String topoJsonText = MyResources.INSTANCE.topojsonEarth().getText();
// creates a topoJSON object, needed for further computations
TopoJson world = GeoUtil.createTopoJson(topoJsonText);
Features
A topology is a TopoJSON object where the type member’s value is the topology.
A topology must have a member with the name objects
whose value is another object. The value of each member of this object is a geometry object.
Charba provides methods, by GeoUtil, to parse the TopoJSON definition and provide a list of Features which are mapping the topology needed to render in the chart.
Every TopoJSON definition object could be built with a different name spaces, therefore the node name to use as root of object must be provided by the developer.
// gets the topoJSON map as string
String topoJsonText = MyResources.INSTANCE.topojsonEarth().getText();
// creates a topoJSON object, needed for further computations
TopoJson world = GeoUtil.createTopoJson(topoJsonText);
// the node name in "objects" one where the topology
// are stored is "countries"
List<Feature> features = GeoUtil.features(world, "countries");
A Feature contains the properties of the topology.
Even in this case, the properties names are not standard. Every feature can have own property names.
// defines the property name "name"
private static final Key NAME = Key.create("name");
...
// gets the topoJSON map as string
String topoJsonText = MyResources.INSTANCE.topojsonEarth().getText();
// creates a topoJSON object, needed for further computations
TopoJson world = GeoUtil.createTopoJson(topoJsonText);
// the node name in "objects" one where the topology
// are stored is "countries"
List<Feature> features = GeoUtil.features(world, "countries");
for (Feature feature: features) {
// gets the name of the country by NAME key instance
String countryName = feature.getStringProperty(NAME);
...
}
Labels
Usually the labels to display for the geographic map chart are stored in the properties of a Feature, for instance the name of the country, state, regions, provinces, cities and so on. As said above, the properties names are not fixed for all topologies and every topology can have own properties.
To improve to the access of topology definitions and to help the labels creation, Charba provides methods, by GeoUtil, which creates a Labels to set to the chart, as following:
// defines the property name "name"
private static final Key NAME = Key.create("name");
...
// gets the topoJSON map as string
String topoJsonText = MyResources.INSTANCE.topojsonEarth().getText();
// creates a topoJSON object, needed for further computations
TopoJson world = GeoUtil.createTopoJson(topoJsonText);
// the node name in "objects" one where the topology
// are stored is "countries"
List<Feature> features = GeoUtil.features(world, "countries");
// creates a labels object with all countries names.
Labels labels = GeoUtil.loadLabels(features, NAME);
// sets labels to chart
chart.getData().setLabels(labels);
The labels enables the tooltip to shows the property related to the topology that you are representing.
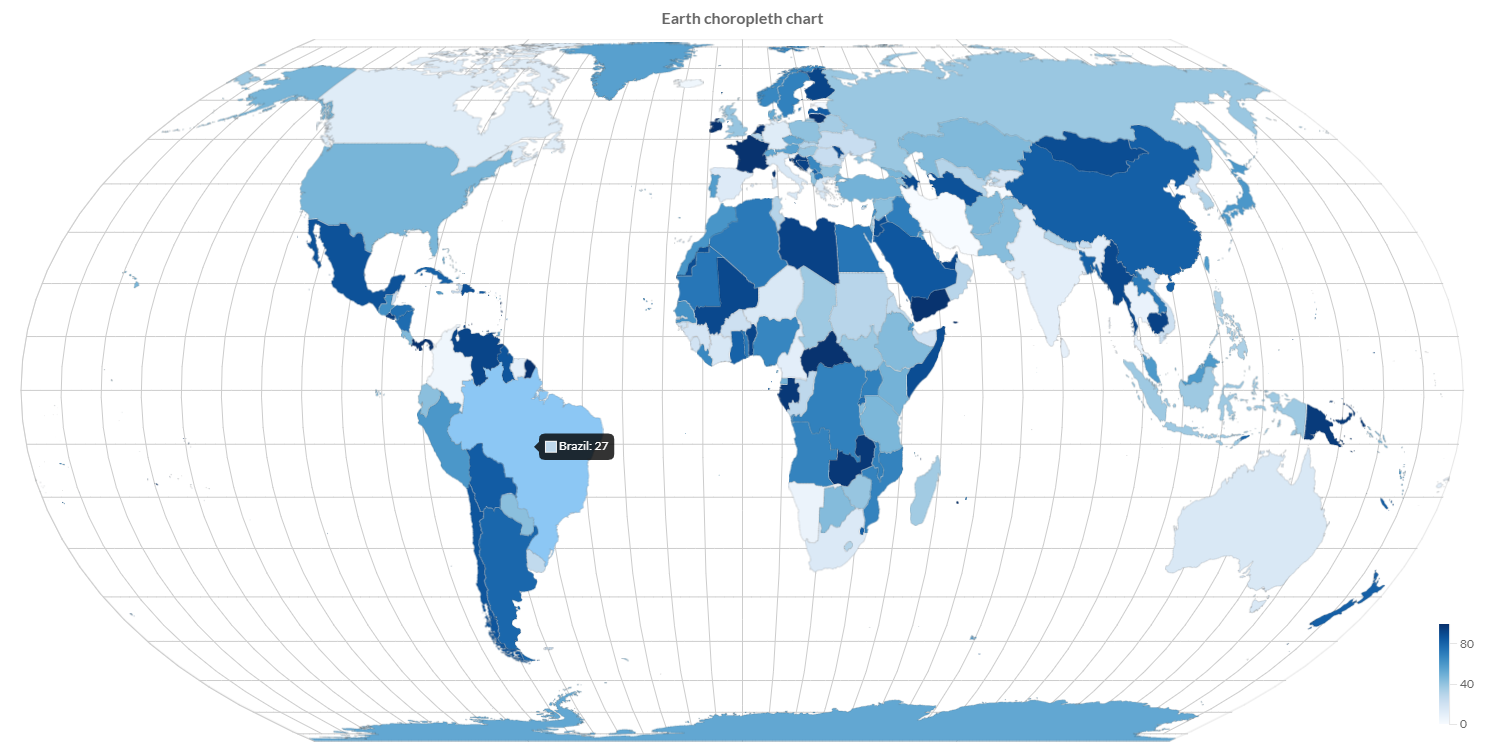